The Assignment class is used to store an explicit equation. More...
#include <Assignment.h>
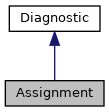
Public Member Functions | |
Assignment (Value &Lhs, const Value &Rhs, std::string tag) | |
~Assignment (void) | |
Destructor. More... | |
double | delta (void) const |
Computes absolute value of relative error for the difference between RHS and LHS. More... | |
double | rhs (void) const |
returns the current numeric value of the rhs More... | |
double | lhs (void) const |
returns the current numeric value of the lhs More... | |
void | directstep (double damping, double maxChange) |
void | wegsteinstep (void) |
Updates *LHSP with RHS using Wegstein accelerated direct substitution. More... | |
std::ostream & | print (std::ostream &) const |
void | printme (void) const |
![]() | |
Diagnostic (void) | |
void | setVerbosity (int verbosity) |
sets the verbosity level for this instance More... | |
int | verbosity (void) const |
Public Attributes | |
std::string | TAG |
Descriptive tag for the Assignment. More... | |
Value * | LHSP |
holds a pointer to the left hands side to make it possible to manipulate it with Assignment::directstep() or Assignment::wegsteinstep(). More... | |
Value | RHS |
holds the right hand side value after the last execution of assembly containing this Assignment. More... | |
Value | oldLHS |
stores the value of the left hand side before the last Assignment::directstep() or Assignment::wegsteinstep(). More... | |
Value | oldRHS |
stores the value of the left hand side before the last execution of the assembly. More... | |
Value | factor |
residual will be multiplied by this factor when solving simultaneously More... | |
std::string | RHSS |
holds the right hand side string More... | |
Friends | |
std::ostream & | operator<< (std::ostream &os, const Assignment &e) |
Additional Inherited Members | |
![]() | |
virtual | ~Diagnostic ()=default |
![]() | |
int | verbosityInstance |
Detailed Description
The Assignment class is used to store an explicit equation.
Explicit equations are those which can be written in the form: y = f(...) where y is an lvalue and f(...) is any function. The evaluation of the right hand side is performed by the assembly containing this Assignment.
Constructor & Destructor Documentation
◆ Assignment()
Constructor
- Parameters
-
Lhs the left hand side, a Value lvalue where the result of the right hand side should be written Rhs the right hand side tag the descriptive tag
◆ ~Assignment()
Assignment::~Assignment | ( | void | ) |
Destructor.
Member Function Documentation
◆ delta()
double Assignment::delta | ( | void | ) | const |
Computes absolute value of relative error for the difference between RHS and LHS.
◆ directstep()
void Assignment::directstep | ( | double | damping, |
double | maxChange | ||
) |
Substitutes RHS into *LHSP
- Parameters
-
damping damping coefficient maxChange maximum relative step change
◆ lhs()
double Assignment::lhs | ( | void | ) | const |
returns the current numeric value of the lhs
◆ print()
std::ostream & Assignment::print | ( | std::ostream & | ) | const |
◆ printme()
void Assignment::printme | ( | void | ) | const |
◆ rhs()
double Assignment::rhs | ( | void | ) | const |
returns the current numeric value of the rhs
◆ wegsteinstep()
void Assignment::wegsteinstep | ( | void | ) |
Updates *LHSP with RHS using Wegstein accelerated direct substitution.
Friends And Related Function Documentation
◆ operator<<
|
friend |
Member Data Documentation
◆ factor
Value Assignment::factor |
residual will be multiplied by this factor when solving simultaneously
◆ LHSP
Value* Assignment::LHSP |
holds a pointer to the left hands side to make it possible to manipulate it with Assignment::directstep() or Assignment::wegsteinstep().
◆ oldLHS
Value Assignment::oldLHS |
stores the value of the left hand side before the last Assignment::directstep() or Assignment::wegsteinstep().
◆ oldRHS
Value Assignment::oldRHS |
stores the value of the left hand side before the last execution of the assembly.
◆ RHS
Value Assignment::RHS |
holds the right hand side value after the last execution of assembly containing this Assignment.
◆ RHSS
std::string Assignment::RHSS |
holds the right hand side string
◆ TAG
std::string Assignment::TAG |
Descriptive tag for the Assignment.
The documentation for this class was generated from the following file: