Model for physical quantities complete with unit of measurement, derivates and persistency. More...
#include <Value.h>
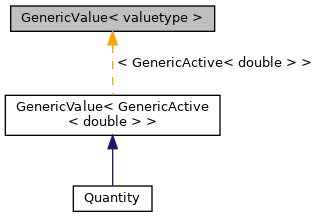
Public Member Functions | |
GenericValue (void) | |
Default constructor. More... | |
GenericValue (const GenericValue< valuetype > &) | |
Copy constructor (this class is copyable) More... | |
GenericValue (double) | |
Value-only constructor (no UOM) More... | |
GenericValue (double v, const std::string s) | |
GenericValue (const std::string) | |
UOM-only constructor (no value) More... | |
GenericValue (double v, const UnitArray u) | |
GenericValue (GenericActive< double > v, const std::string s="") | |
GenericValue (GenericActive< double > v, const UnitArray u) | |
virtual | ~GenericValue (void) |
const valuetype & | value (void) const |
valuetype & | value_rw (void) |
double | toDouble (void) const |
double | toDouble (const std::string s) const |
returns a new object with unchanged value but zero derivatives More... | |
GenericValue< valuetype > | inactivated (void) const |
GenericValue< valuetype > | operator- (void) const |
template<class rhstype > | |
GenericValue< valuetype > | operator+ (const rhstype &rhs) const |
GenericValue< valuetype > | operator+ (const GenericValue< valuetype > &) const |
template<class rhstype > | |
GenericValue< valuetype > | operator- (const rhstype &rhs) const |
GenericValue< valuetype > | operator- (const GenericValue< valuetype > &rhs) const |
template<class rhstype > | |
GenericValue< valuetype > | operator* (const rhstype &rhs) const |
template<class rhstype > | |
GenericValue< valuetype > | operator/ (const rhstype &rhs) const |
bool | operator!= (const GenericValue< valuetype > &) const |
bool | operator== (const GenericValue< valuetype > &) const |
bool | operator<= (const GenericValue< valuetype > &) const |
bool | operator>= (const GenericValue< valuetype > &) const |
bool | operator< (const GenericValue< valuetype > &) const |
bool | operator> (const GenericValue< valuetype > &) const |
GenericValue< valuetype > & | operator= (double v) |
GenericValue< valuetype > & | operator= (valuetype v) |
GenericValue< valuetype > & | operator= (const GenericValue< valuetype > &a) |
GenericValue< valuetype > & | operator+= (double v) |
GenericValue< valuetype > & | operator+= (valuetype v) |
GenericValue< valuetype > & | operator+= (const GenericValue< valuetype > &a) |
GenericValue< valuetype > & | operator-= (double v) |
GenericValue< valuetype > & | operator-= (valuetype v) |
GenericValue< valuetype > & | operator-= (const GenericValue< valuetype > &a) |
GenericValue< valuetype > & | operator*= (double rhs) |
GenericValue< valuetype > & | operator*= (valuetype rhs) |
GenericValue< valuetype > & | operator*= (const GenericValue< valuetype > &a) |
GenericValue< valuetype > & | operator/= (double rhs) |
GenericValue< valuetype > & | operator/= (valuetype rhs) |
GenericValue< valuetype > & | operator/= (const GenericValue< valuetype > &a) |
void | clear (void) |
set to zero More... | |
double | checkuom (const std::string &s) const |
const bool & | input (void) const |
const bool & | output (void) const |
void | setOutput (void) |
Set the GenericValue as output parameter of a model. More... | |
void | setInput (void) |
Set the GenericValue as input parameter of a model. More... | |
void | unSetOutput (void) |
Reset the GenericValue as default no-output parameter of a model. More... | |
void | unSetInput (void) |
Reset the GenericValue as default no-input parameter of a model. More... | |
void | set (double v, const std::string s="") |
void | set (GenericValue< valuetype >) |
Sets the GenericValue copying another GenericValue. More... | |
void | set (valuetype) |
Sets the GenericValue only with valuetype. More... | |
const UnitArray & | unit (void) const |
Returns the UnitArray of UOM. More... | |
void | divideUnit (int factor) |
divide all exponents of the unit by factor; used in sqrt and curt More... | |
void | forceUnit (const UnitArray &uom) |
Sets the unit of measurement (not recomended: use a proper constructor or setter) More... | |
std::string | getUnit (void) const |
Returns the UOM as string. More... | |
std::string | getUnittovector (void) const |
Returns the UOM as string. More... | |
Friends | |
std::ostream & | operator<< (std::ostream &os, const GenericValue< valuetype > &quantity) |
Detailed Description
class GenericValue< valuetype >
Model for physical quantities complete with unit of measurement, derivates and persistency.
Supported operations:
- - negative value
- + addition (left and right operator) with valuetype, GenericValue<valuetype>, GenericActive<valuetype>
- - substraction (left and right operator) with valuetype, GenericValue<valuetype>, GenericActive<valuetype>
- * multiplication (left and right operator) with valuetype, GenericValue<valuetype>, GenericActive<valuetype>
- / division (left and right operator) with valuetype, GenericValue<valuetype>, GenericActive<valuetype>
- comparisons (!= , == , <= , >= , < , >) between GenericValue<valuetype>
- functions between GenericValue<valuetype>:
- exp: exponential
- log: natural logarithm
- log10: 10-base logarithm
- sin: sine
- asin: arc sine
- tan: tangent
- atan: arc tangent
- cos: cosine
- acos: arc cosine
- sinh: hyperbolic sine
- cosh: hyperbolic cosine
- sqrt: square root
- curt: cubic root
- pow: power
- fabs: absolute value
- = assignment of valuetype and GenericValue (assignement constructor)
- += addition assignment with valuetype, GenericValue<valuetype>, GenericActive<valuetype>
- -= substraction assignment with valuetype, GenericValue<valuetype>, GenericActive<valuetype>
- *= multiplication assignment with valuetype, GenericValue<valuetype>
- /= division assignment with valuetype, GenericValue<valuetype>
#include <libpf/value/Value.h>
- Remarks
- Actually supported GenericValue< double > (typedef Value)
- Warning
- Thread safe:
- Extendable:
- Platform dependencies:
Constructor & Destructor Documentation
◆ GenericValue() [1/8]
GenericValue< valuetype >::GenericValue | ( | void | ) |
Default constructor.
◆ GenericValue() [2/8]
GenericValue< valuetype >::GenericValue | ( | const GenericValue< valuetype > & | ) |
Copy constructor (this class is copyable)
◆ GenericValue() [3/8]
|
explicit |
Value-only constructor (no UOM)
◆ GenericValue() [4/8]
GenericValue< valuetype >::GenericValue | ( | double | v, |
const std::string | s | ||
) |
Suggested constructor
- Parameters
-
v value s Unit of measurement string
◆ GenericValue() [5/8]
|
explicit |
UOM-only constructor (no value)
◆ GenericValue() [6/8]
|
explicit |
Constructor passing UOM as UnitArray
- Parameters
-
v value u Unit of measurement UnitArray
◆ GenericValue() [7/8]
|
explicit |
Constructor passing GenericActive value
- Parameters
-
v value as GenericActive s Unit of measurement string
◆ GenericValue() [8/8]
|
explicit |
Constructor passing GenericActive value and UOM as UnitArray
- Parameters
-
v value u Unit of measurement UnitArray
◆ ~GenericValue()
|
virtual |
Member Function Documentation
◆ checkuom()
double GenericValue< valuetype >::checkuom | ( | const std::string & | s | ) | const |
Check existance and correctness of UOM
- Parameters
-
s UOM string
◆ clear()
void GenericValue< valuetype >::clear | ( | void | ) |
set to zero
◆ divideUnit()
|
inline |
divide all exponents of the unit by factor; used in sqrt and curt
◆ forceUnit()
void GenericValue< valuetype >::forceUnit | ( | const UnitArray & | uom | ) |
Sets the unit of measurement (not recomended: use a proper constructor or setter)
◆ getUnit()
std::string GenericValue< valuetype >::getUnit | ( | void | ) | const |
Returns the UOM as string.
◆ getUnittovector()
std::string GenericValue< valuetype >::getUnittovector | ( | void | ) | const |
Returns the UOM as string.
◆ inactivated()
GenericValue< valuetype > GenericValue< valuetype >::inactivated | ( | void | ) | const |
◆ input()
const bool & GenericValue< valuetype >::input | ( | void | ) | const |
Return the GenericValue as input status
- Returns
- a const reference to the GenericValue input status
◆ operator!=()
bool GenericValue< valuetype >::operator!= | ( | const GenericValue< valuetype > & | ) | const |
◆ operator*()
GenericValue< valuetype > GenericValue< valuetype >::operator* | ( | const rhstype & | rhs | ) | const |
◆ operator*=() [1/3]
GenericValue< valuetype > & GenericValue< valuetype >::operator*= | ( | const GenericValue< valuetype > & | a | ) |
◆ operator*=() [2/3]
GenericValue< valuetype > & GenericValue< valuetype >::operator*= | ( | double | rhs | ) |
◆ operator*=() [3/3]
GenericValue< valuetype > & GenericValue< valuetype >::operator*= | ( | valuetype | rhs | ) |
◆ operator+() [1/2]
GenericValue< valuetype > GenericValue< valuetype >::operator+ | ( | const GenericValue< valuetype > & | ) | const |
◆ operator+() [2/2]
GenericValue< valuetype > GenericValue< valuetype >::operator+ | ( | const rhstype & | rhs | ) | const |
◆ operator+=() [1/3]
GenericValue< valuetype > & GenericValue< valuetype >::operator+= | ( | const GenericValue< valuetype > & | a | ) |
◆ operator+=() [2/3]
GenericValue< valuetype > & GenericValue< valuetype >::operator+= | ( | double | v | ) |
◆ operator+=() [3/3]
GenericValue< valuetype > & GenericValue< valuetype >::operator+= | ( | valuetype | v | ) |
◆ operator-() [1/3]
GenericValue< valuetype > GenericValue< valuetype >::operator- | ( | const GenericValue< valuetype > & | rhs | ) | const |
◆ operator-() [2/3]
GenericValue< valuetype > GenericValue< valuetype >::operator- | ( | const rhstype & | rhs | ) | const |
◆ operator-() [3/3]
GenericValue< valuetype > GenericValue< valuetype >::operator- | ( | void | ) | const |
◆ operator-=() [1/3]
GenericValue< valuetype > & GenericValue< valuetype >::operator-= | ( | const GenericValue< valuetype > & | a | ) |
◆ operator-=() [2/3]
GenericValue< valuetype > & GenericValue< valuetype >::operator-= | ( | double | v | ) |
◆ operator-=() [3/3]
GenericValue< valuetype > & GenericValue< valuetype >::operator-= | ( | valuetype | v | ) |
◆ operator/()
GenericValue< valuetype > GenericValue< valuetype >::operator/ | ( | const rhstype & | rhs | ) | const |
◆ operator/=() [1/3]
GenericValue< valuetype > & GenericValue< valuetype >::operator/= | ( | const GenericValue< valuetype > & | a | ) |
◆ operator/=() [2/3]
GenericValue< valuetype > & GenericValue< valuetype >::operator/= | ( | double | rhs | ) |
◆ operator/=() [3/3]
GenericValue< valuetype > & GenericValue< valuetype >::operator/= | ( | valuetype | rhs | ) |
◆ operator<()
bool GenericValue< valuetype >::operator< | ( | const GenericValue< valuetype > & | ) | const |
◆ operator<=()
bool GenericValue< valuetype >::operator<= | ( | const GenericValue< valuetype > & | ) | const |
◆ operator=() [1/3]
GenericValue< valuetype > & GenericValue< valuetype >::operator= | ( | const GenericValue< valuetype > & | a | ) |
◆ operator=() [2/3]
GenericValue< valuetype > & GenericValue< valuetype >::operator= | ( | double | v | ) |
◆ operator=() [3/3]
GenericValue< valuetype > & GenericValue< valuetype >::operator= | ( | valuetype | v | ) |
◆ operator==()
bool GenericValue< valuetype >::operator== | ( | const GenericValue< valuetype > & | ) | const |
◆ operator>()
bool GenericValue< valuetype >::operator> | ( | const GenericValue< valuetype > & | ) | const |
◆ operator>=()
bool GenericValue< valuetype >::operator>= | ( | const GenericValue< valuetype > & | ) | const |
◆ output()
const bool & GenericValue< valuetype >::output | ( | void | ) | const |
- Returns
- a const reference to the GenericValue output status
◆ set() [1/3]
void GenericValue< valuetype >::set | ( | double | v, |
const std::string | s = "" |
||
) |
Sets the GenericValue (recomended setter)
- Parameters
-
v value s unit of measurement
◆ set() [2/3]
void GenericValue< valuetype >::set | ( | GenericValue< valuetype > | ) |
Sets the GenericValue copying another GenericValue.
◆ set() [3/3]
void GenericValue< valuetype >::set | ( | valuetype | ) |
Sets the GenericValue only with valuetype.
◆ setInput()
void GenericValue< valuetype >::setInput | ( | void | ) |
Set the GenericValue as input parameter of a model.
◆ setOutput()
void GenericValue< valuetype >::setOutput | ( | void | ) |
Set the GenericValue as output parameter of a model.
◆ toDouble() [1/2]
double GenericValue< valuetype >::toDouble | ( | const std::string | s | ) | const |
returns a new object with unchanged value but zero derivatives
Returns the value with generic UOM
- Parameters
-
s generic UOM
◆ toDouble() [2/2]
double GenericValue< valuetype >::toDouble | ( | void | ) | const |
- Returns
- underlying value as a double
◆ unit()
|
inline |
Returns the UnitArray of UOM.
◆ unSetInput()
void GenericValue< valuetype >::unSetInput | ( | void | ) |
Reset the GenericValue as default no-input parameter of a model.
◆ unSetOutput()
void GenericValue< valuetype >::unSetOutput | ( | void | ) |
Reset the GenericValue as default no-output parameter of a model.
◆ value()
const valuetype & GenericValue< valuetype >::value | ( | void | ) | const |
- Returns
- read-only underlying value
◆ value_rw()
valuetype & GenericValue< valuetype >::value_rw | ( | void | ) |
- Returns
- read-write underlying value
Friends And Related Function Documentation
◆ operator<<
|
friend |
The documentation for this class was generated from the following file: