#include <Model.h>
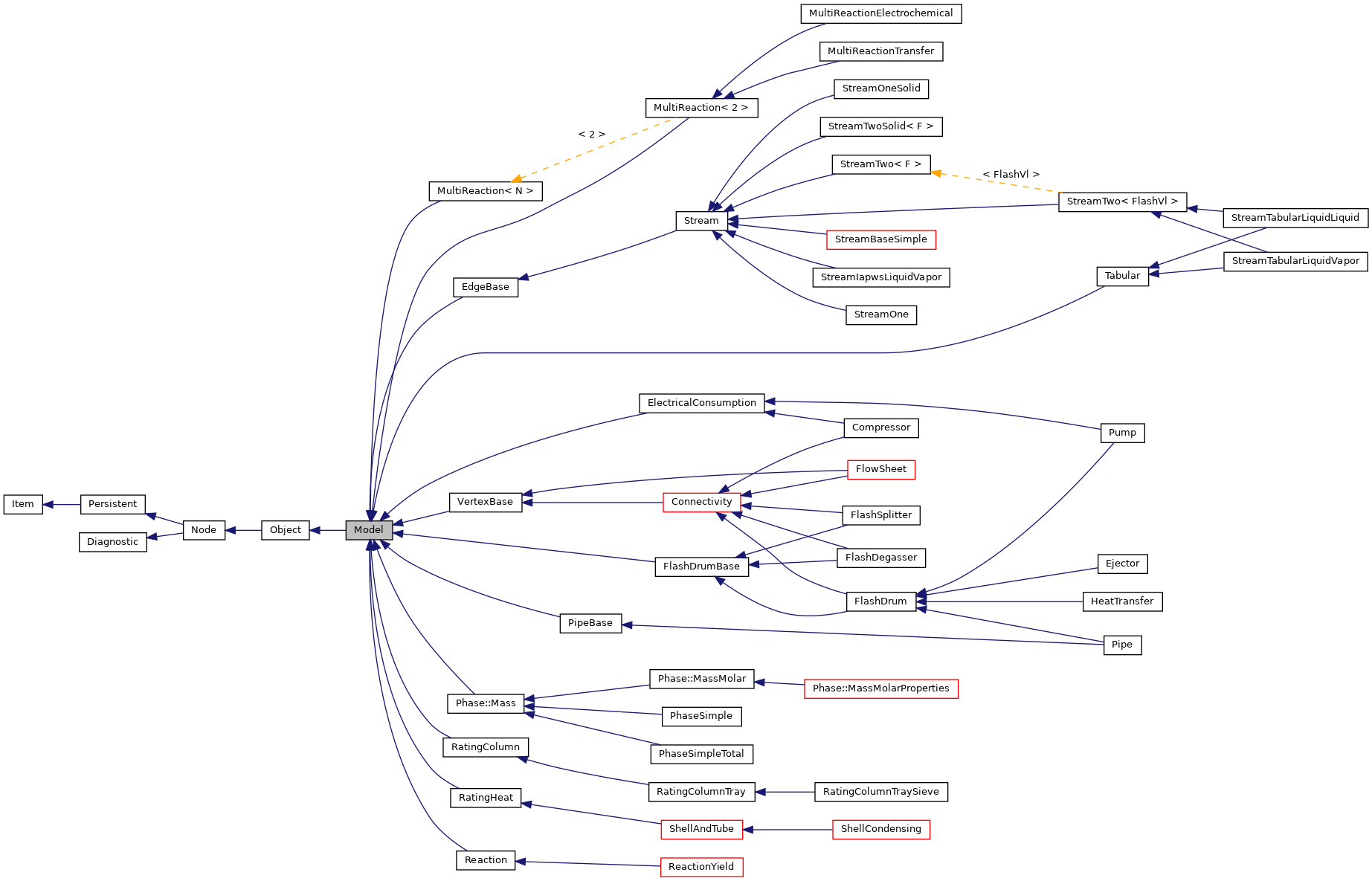
Public Member Functions | |
Model (Libpf::Persistency::Defaults defaults, uint32_t id, Persistency *persistency, Persistent *parent, Persistent *root) | |
INTEGER (nCalculations, "Number of times the model has been calculated", 0) | |
STRINGVECTOR (errors, "Errors from the last computation", 0, "") | |
STRINGVECTOR (warnings, "Warnings from the last computation", 0, "") | |
bool | isPristine (void) const |
void | unSetInput (void) |
wipes out all tags marked as inputs More... | |
void | reportMessages (void) |
recursively collect status of all sub-objects and report to parent More... | |
void | resetErrors (void) |
void | setWarning (std::string s) |
send new warning message to local queue More... | |
void | setError (std::string s) |
send new error message to local queue More... | |
void | reportWarning (std::string s) |
propagate warning message from sub-object to local queue More... | |
void | reportError (std::string s) |
propagate error message from sub-object to local queue More... | |
void | setCalculated (void) |
increments nCalculations counter and unsets pristinity More... | |
void | setPristineRecursive (void) |
force next calculation to restart as if the model had never been calculated during this runtime session, also for all contained objects More... | |
void | setPristine (void) |
force next calculation to restart as if the model had never been calculated during this runtime session More... | |
void | setRunTime (double t) |
set the run time More... | |
virtual Libpf::Utility::Category | category (void) const =0 |
return model Category More... | |
virtual void | calculate (int level=0)=0 |
virtual void | setup (void)=0 |
performs initializations required immediately before solution More... | |
virtual int | maximumIterations (void) |
returns default maximum iterations for main computation; defaults to 200 More... | |
virtual void | initializeNonPersistents (void)=0 |
estimates hidden, non-persistent unknowns from the current results More... | |
virtual ObjectiveNleAd * | objectiveFunction (void)=0 |
returns a pointer to the model's objective function More... | |
std::ostream & | print (std::ostream &os, bool strings, bool quantities, bool integers, bool recursive) const |
std::ostream & | printFull (std::ostream &) const |
print the model quantities, including derivative info, in text format to os More... | |
void | printHtml (const char *dir) const |
virtual void | printSvg (const char *fileName, bool tiny, bool raster, int offset) |
void | printSvgAll (const char *dir, bool tiny, bool raster, int offset, std::vector< std::string > &list) |
Recursively output the model and all descendants in SVG format as separate id.SVG files in the directory dir. More... | |
virtual void | printXml (const char *dir, Model *precedingSibling, Model *followingSibling) const |
void | printXmlToc (std::ofstream &toc, int indent) const |
void | printOds (std::ofstream &ods) const |
![]() | |
Object (Libpf::Persistency::Defaults defaults, uint32_t id=0, Persistency *persistency=nullptr, Persistent *parent=nullptr, Persistent *root=nullptr) | |
Libpf::Persistency::Defaults | defaults (void) const |
std::ostream & | printJson (std::ostream &os, int level=0) const override |
print Node in JSON format More... | |
void | readVariables (Persistency *persistency) override |
reads Q, QV and QM from persistency More... | |
void | readParameters (Persistency *persistency) override |
reads I, IV, S and SV from persistency More... | |
Node & | operator= (const Node &) override |
copy assignment operator More... | |
Node & | operator= (Node &&other) override |
move assignment More... | |
~Object (void) | |
const std::string & | type (void) const override |
Object & | at (const std::string &fullRelativeTag) |
const Object & | at (const std::string &fullRelativeTag) const |
Object & | at (const std::string &fullRelativeTag, int index) |
const Value & | Q (const std::string &tag) const |
const Value & | Q (const std::string &tag, int index) const |
const Value & | Q (const std::string &tag, int row, int column) const |
Value & | Q (const std::string &tag) |
Value & | Q (const std::string &tag, int index) |
Value & | Q (const std::string &tag, int row, int column) |
const Value & | Q (const std::string &tag, std::string componentName) const |
Value & | Q (const std::string &tag, std::string componentName) |
const Value & | Q (const std::string &tag, int index, std::string componentName) const |
Value & | Q (const std::string &tag, int index, std::string componentName) |
const int & | I (const std::string &tag) const |
const int & | I (const std::string &tag, int index) const |
int & | I (const std::string &tag) |
int & | I (const std::string &tag, int index) |
const std::string & | S (const std::string &tag) const |
const std::string & | S (const std::string &tag, int index) const |
std::string & | S (const std::string &tag) |
std::string & | S (const std::string &tag, int index) |
bool | operator!= (const Object &rhs) const |
bool | operator== (const Object &rhs) const |
bool | compareParameters (const Object &rhs) const |
bool | existsI (const std::string &tag) const |
bool | existsS (const std::string &tag) const |
bool | existsQ (const std::string &tag) const |
void | push (void) const |
store the current status of the object and all its sub-objects in the last-in-first-out queue of real variables values More... | |
void | pop (void) |
restore the status of the object and all its sub-objects from the last-in-first-out queue of real variables values More... | |
void | clear (void) const |
clear the last-in-first-out queue of real variables values used for storing and retrieving the status More... | |
const RangeQuantities | quantities (void) |
const ConstRangeQuantities | quantities (void) const |
![]() | |
Node (Libpf::Persistency::Defaults defaults, uint32_t id=0, Persistency *persistency=nullptr, Persistent *parent=nullptr, Persistent *root=nullptr) | |
Node (const Node &other) | |
copy constructor More... | |
Node (Node &&other) | |
move constructor More... | |
virtual std::unique_ptr< Node > | clone (void) const |
virtual Node & | operator= (const Node &other) |
copy assignment operator copies all elements but root which is set to nullptr More... | |
virtual Node & | operator= (Node &&other) |
move assignment More... | |
~Node () | |
int | insert (Persistency *persistency) const override |
int | update (Persistency *persistency) const override |
void | restore (Persistency *persistency) override |
void | remove (Persistency *persistency) const override |
uint32_t | rootId (void) const override |
uint32_t | range (void) const override |
uint32_t | getId (void) override |
returns the next available id and increments the internal counter More... | |
const std::string & | type (void) const override |
virtual std::ostream & | printJson (std::ostream &os, int level=0) const |
print Node in JSON format More... | |
Node & | addChild (std::string type, uint32_t id, Persistency *persistency) |
Node & | addChild (std::string type, Libpf::Persistency::Defaults defaults) |
Node & | addChild (const Node &child) |
Node & | addChild (std::unique_ptr< Node > child) |
std::unique_ptr< Node > | prune (const std::string &tag) |
const Node & | child (const std::string &tag) const |
Node & | at (const std::string &fullRelativeTag) |
const Node & | at (const std::string &fullRelativeTag) const |
uint32_t | descendants (void) const |
Persistent * | root (void) const |
void | renameChild (const std::string &oldName, const std::string &newName) |
bool | existsChild (std::string tag) const |
bool | exists (uint32_t id) |
Node & | search (uint32_t id) |
bool | operator!= (const Node &rhs) const |
bool | operator== (const Node &rhs) const |
virtual void | readVariables (Persistency *) |
reads Q, QV and QM from persistency More... | |
virtual void | readParameters (Persistency *) |
reads I, IV, S and SV from persistency More... | |
bool | isRestored (void) const |
return whether the Node has just been retrieved from persistent storage More... | |
void | setIcon (std::string icon, double width, double height) |
bool | hasIcon (void) const |
std::string | iconName (bool raster) const |
double | iconWidth (void) const |
double | iconHeight (void) const |
const Range | children (void) |
const ConstRange | children (void) const |
![]() | |
Persistent (const std::string &tag, const std::string &description, Persistent *parent, uint32_t id) | |
main constructor More... | |
Persistent (const Persistent &) | |
copy constructor More... | |
Persistent & | operator= (const Persistent &) |
copy assignment More... | |
Persistent (Persistent &&other) | |
move constructor More... | |
Persistent & | operator= (Persistent &&other) |
move assignment More... | |
~Persistent (void) | |
uint32_t | id (void) const |
std::string | uuid (void) const |
uint32_t | parentId (void) const |
virtual uint32_t | rootId (void) const =0 |
virtual uint32_t | range (void) const =0 |
virtual uint32_t | getId (void)=0 |
returns the next available id and increments the internal counter More... | |
double | created_at (void) const |
double | updated_at (void) const |
void | updated_at (double u) const |
virtual int | insert (Persistency *persistency) const =0 |
virtual int | update (Persistency *persistency) const =0 |
virtual void | restore (Persistency *persistency)=0 |
virtual void | remove (Persistency *persistency) const =0 |
![]() | |
Item (const Item &other) | |
copy constructor copies all elements but parent which is set to nullptr More... | |
Item & | operator= (const Item &other) |
copy assignment operator copies all elements but parent which is set to nullptr More... | |
Item (const std::string &tag, const std::string &description, Persistent *parent) | |
Item (Item &&other) | |
move constructor More... | |
Item & | operator= (Item &&other) |
move assignment operator More... | |
virtual | ~Item () |
const std::string & | tag () const |
const std::string & | description () const |
std::string | fullTag () const |
const Persistent * | parent () const |
virtual const std::string & | type () const =0 |
void | setTag (const std::string &tag) |
void | setDescription (const std::string &description) |
void | setWideTag (const std::wstring &tag) |
wide-character variant, More... | |
void | setWideDescription (const std::wstring &description) |
wide-character variant, More... | |
bool | operator!= (const Item &rhs) const |
bool | operator== (const Item &rhs) const |
![]() | |
Diagnostic (void) | |
void | setVerbosity (int verbosity) |
sets the verbosity level for this instance More... | |
int | verbosity (void) const |
Protected Member Functions | |
void | printSvgXlink (std::ostream &svg, const std::string &link, double width, double height) |
void | addComponentVectorVariable (std::vector< Quantity > &variable, const std::string &tag, const std::string &description, Quantity::ValueType value) |
template<typename T > | |
void | addSubObjects (int verbosityFile, Libpf::Persistency::Defaults defaults, Persistency *persistency, int count, const std::string &optionKey, const std::string &typeKey, const std::string &tagKey, const std::string &description, std::vector< T * > &vector) |
![]() | |
int | retrieveInteger (const Libpf::Persistency::Defaults &defaults, uint32_t id, Persistency *persistency, std::string tag, int min, int max, int def=-1) |
std::string | retrieveString (const Libpf::Persistency::Defaults &defaults, uint32_t id, Persistency *persistency, std::string tag, const std::string def) |
template<class T > | |
void | addVariable (T &variable) |
template<class T > | |
void | addVectorVariable (std::vector< T > &variable, const std::string &tag, const std::string &description, uint32_t size, typename T::ValueType value) |
Object (const Object &other) | |
copy constructor More... | |
Object (Object &&other) | |
move constructor More... | |
Object & | operator= (const Object &) |
copy assignment operator More... | |
Object & | operator= (Object &&other) |
move assignment operator More... | |
![]() | |
int | offset (void) const |
![]() | |
virtual | ~Diagnostic ()=default |
Additional Inherited Members | |
![]() | |
std::map< std::string, Integer * > | integers_ |
collection of integer variables More... | |
std::map< std::string, IntegerVector * > | integerVectors_ |
collection of integer variable vectors More... | |
std::map< std::string, Quantity * > | quantities_ |
collection of real variables More... | |
std::map< std::string, QuantityVector * > | quantityVectors_ |
collection of real variable vectors More... | |
std::map< std::string, QuantityMatrix * > | quantityMatrices_ |
collection of real variable matrices More... | |
std::map< std::string, String * > | strings_ |
collection of string variables More... | |
std::map< std::string, StringVector * > | stringVectors_ |
collection of string variable vectors More... | |
![]() | |
std::map< std::string, std::unique_ptr< Node > > | children_ |
the collection of direct descendants More... | |
![]() | |
Persistent * | parent_ |
pointer to the parent Persistent; set to this by the constructor if Item is self-standing or a root Persistent of a tree More... | |
![]() | |
int | verbosityInstance |
Detailed Description
extends Object to provide:
- output (printXml, printSvg...)
- calculation (calculate...) facilities
Constructor & Destructor Documentation
◆ Model()
Model::Model | ( | Libpf::Persistency::Defaults | defaults, |
uint32_t | id, | ||
Persistency * | persistency, | ||
Persistent * | parent, | ||
Persistent * | root | ||
) |
Member Function Documentation
◆ addComponentVectorVariable()
|
protected |
populates a vector variable indexed by components and inserts the references in the collections of variables
- Parameters
-
variable the vector variable tag the tag of the vector variable description the description of the vector variable value the initialization value for the elements of the vector variable
◆ addSubObjects()
|
protected |
populates a vector of sub-objects and registers them as children of the Node
- Exceptions
-
ErrorRunTime if the sub-object type is unspecified
- Parameters
-
[in] verbosityFile the file-level verbosity [in] defaults default informations to build and configure the sub-objects [in] persistency database connection [in] count number of sub-objects [in] optionKey base string to find the sub-object types in the default data structure [in] typeKey base string to recognize the sub-object types [in] tagKey base string to generate the tags [in] description UTF-8 encoded description [out] vector the sub-object pointers will be placed in here; the vector will be resized if necessary
◆ calculate()
|
pure virtual |
calculates the model; implementations must call setCalculated
- Parameters
-
level used for indenting, defaults to 0
Implemented in FlowSheet, RatingColumnTraySieve, Terminator, Phase::Mass, Phase::MassMolar, Stream, Compressor, Degasser, Divider, Exchanger, FlashDegasser, FlashDrum, FlashSplitter, HtuNtu, Mixer, MultiExchanger, Multiplier, MultiReaction< N >, MultiReaction< 2 >, MultiReactionTransfer, Pump, RatingHeat, ReactionYield, Selector, Separator, and Splitter.
◆ category()
|
pure virtual |
return model Category
Implemented in EdgeBase, VertexBase, FlowSheet, RatingColumn, Phase::Mass, MultiReaction< N >, MultiReaction< 2 >, RatingHeat, and Reaction.
◆ initializeNonPersistents()
|
pure virtual |
estimates hidden, non-persistent unknowns from the current results
Implemented in FlowSheet, RatingColumnTraySieve, Terminator, PhaseGeneric< T >, PhaseGeneric< U >, PhaseSimple, PhaseSimpleTotal, PhaseTotal, Stream, StreamIapwsLiquidVapor, StreamTwo< F >, StreamTwo< FlashVl >, StreamTwoSolid< F >, Compressor, Degasser, Divider, Exchanger, FlashDegasser, FlashDrum, FlashSplitter, HtuNtu, Mixer, MultiExchanger, Multiplier, MultiReaction< N >, MultiReaction< 2 >, RatingHeat, Reaction, Selector, Separator, and Splitter.
◆ INTEGER()
Model::INTEGER | ( | nCalculations | , |
"Number of times the model has been calculated" | , | ||
0 | |||
) |
◆ isPristine()
bool Model::isPristine | ( | void | ) | const |
- Returns
- true if the model has not yet been calculated during this runtime session
◆ maximumIterations()
|
virtual |
returns default maximum iterations for main computation; defaults to 200
◆ objectiveFunction()
|
pure virtual |
returns a pointer to the model's objective function
Implemented in FlowSheet, RatingColumnTraySieve, Terminator, Phase::Mass, StreamIapwsLiquidVapor, StreamOne, StreamOneSolid, StreamSimple< NV, NL, NS >, StreamTwo< F >, StreamTwo< FlashVl >, StreamTwoSolid< F >, Compressor, Degasser, Divider, Exchanger, FlashDrumBase, HtuNtu, Mixer, MultiExchanger, Multiplier, MultiReaction< N >, MultiReaction< 2 >, RatingHeat, Reaction, Selector, Separator, and Splitter.
◆ print()
std::ostream & Model::print | ( | std::ostream & | os, |
bool | strings, | ||
bool | quantities, | ||
bool | integers, | ||
bool | recursive | ||
) | const |
print the model in text format to os
- Parameters
-
os the output stream strings turn on output of string variables quantities turn on output of quantity variables integers turn on output of integer variables recursive recursively print sub-models
◆ printFull()
std::ostream & Model::printFull | ( | std::ostream & | ) | const |
print the model quantities, including derivative info, in text format to os
◆ printHtml()
void Model::printHtml | ( | const char * | dir | ) | const |
recursively print the model and all its sub-models as id.html in the directory dir recursively print all nodes as id.html in the directory dir
- Parameters
-
dir the directory where the HTML files will be saved
◆ printOds()
void Model::printOds | ( | std::ofstream & | ods | ) | const |
Output the streams structure in Open Document Format for Office Applications (OpenDocument) v1.1, Spreadsheet document
- Parameters
-
ods file name to write to
◆ printSvg()
|
virtual |
Output the model in SVG format
- Parameters
-
fileName SVG file name (optional full path + file name and .svg extension) tiny if true, print to SVG Tiny 1.1 format, else to Full SVG 1.1 format raster if true, the xlinks in the image elements will point to raster (png) icons, else they will be vector (svg) icons offset offset to apply to the ids
- Remarks
- any flowsheet will be modified afterwards because all cut streams will be reattached and reverted
Reimplemented in VertexBase, and FlowSheet.
◆ printSvgAll()
void Model::printSvgAll | ( | const char * | dir, |
bool | tiny, | ||
bool | raster, | ||
int | offset, | ||
std::vector< std::string > & | list | ||
) |
Recursively output the model and all descendants in SVG format as separate id.SVG files in the directory dir.
- Parameters
-
dir the directory where the SVG files will be saved, without trailing [back]slash tiny if true, print to SVG Tiny 1.1 format, else to Full SVG 1.1 format raster if true, the xlinks in the image elements will point to raster (png) icons, else they will be vector (svg) icons offset offset to apply to the ids [out] list the list of filenames will be appended to this list
- Remarks
- any flowsheet will be modified afterwards because all cut streams will be reattached and reverted
◆ printSvgXlink()
|
protected |
utility function for printSvg, writes to the supplied out svg stream a short SVG file which contains an xlink to the supplied link
- Parameters
-
svg the stream where the SVG should be written to link the filename of the SVG file to link, complete with the SVG extension width the width of the image height the width of the image
◆ printXml()
|
virtual |
recursively print the model and all its sub-models as id.xml in the directory dir
- Parameters
-
dir the directory where the XML files will be saved, without trailing [back]slash precedingSibling set to nullptr if first sibling, else a pointer to the preceding sibling followingSibling set to nullptr if last sibling, else a pointer to the following sibling
◆ printXmlToc()
void Model::printXmlToc | ( | std::ofstream & | toc, |
int | indent | ||
) | const |
prints the table of content as a single xml file
- Parameters
-
toc file name to write to indent number of spaces to indent
◆ reportError()
void Model::reportError | ( | std::string | s | ) |
propagate error message from sub-object to local queue
◆ reportMessages()
void Model::reportMessages | ( | void | ) |
recursively collect status of all sub-objects and report to parent
Delete all error and warning messages and reset to 0 nErrors and nWarnings
◆ reportWarning()
void Model::reportWarning | ( | std::string | s | ) |
propagate warning message from sub-object to local queue
◆ resetErrors()
void Model::resetErrors | ( | void | ) |
◆ setCalculated()
void Model::setCalculated | ( | void | ) |
increments nCalculations counter and unsets pristinity
◆ setError()
void Model::setError | ( | std::string | s | ) |
send new error message to local queue
◆ setPristine()
void Model::setPristine | ( | void | ) |
force next calculation to restart as if the model had never been calculated during this runtime session
◆ setPristineRecursive()
void Model::setPristineRecursive | ( | void | ) |
force next calculation to restart as if the model had never been calculated during this runtime session, also for all contained objects
◆ setRunTime()
void Model::setRunTime | ( | double | t | ) |
set the run time
◆ setup()
|
pure virtual |
performs initializations required immediately before solution
Implemented in Column, ColumnSection, Decanter, FallingFilm, FlowSheet, LiquidRingVacuumPump, CompressionStage, MultiCompressorIntercooled< N >, MultiStage, MultiStageOneTwo, PressureSwingAbsorption, RatingColumnTraySieve, ReboilerCrossFlow, Terminator, Zone, Phase::Mass, Stream, Compressor, Degasser, Divider, ElectricalConsumption, Exchanger, FlashDrum, FlashDrumBase, HeatTransfer, HtuNtu, Mixer, MultiExchanger, Multiplier, MultiReaction< N >, MultiReaction< 2 >, PipeBase, Pipe, Pump, RatingHeat, RatingHeatShellAndTubeFallingFilmReboiler, RatingHeatShellAndTubeHeater, RatingHeatShellAndTubeRecovery, RatingHeatShellAndTubeThermosiphon, ReactionYield, Selector, Separator, ShellAndTube, ShellCondensing, ShellSensible, Splitter, TubesBoiling, TubesFallingFilmBoiling, and TubesSensible.
◆ setWarning()
void Model::setWarning | ( | std::string | s | ) |
send new warning message to local queue
◆ STRINGVECTOR() [1/2]
Model::STRINGVECTOR | ( | errors | , |
"Errors from the last computation" | , | ||
0 | , | ||
"" | |||
) |
◆ STRINGVECTOR() [2/2]
Model::STRINGVECTOR | ( | warnings | , |
"Warnings from the last computation" | , | ||
0 | , | ||
"" | |||
) |
◆ unSetInput()
void Model::unSetInput | ( | void | ) |
wipes out all tags marked as inputs
The documentation for this class was generated from the following file: