Integer Class Reference
integer variable More...
#include <Integer.h>
Inheritance diagram for Integer:
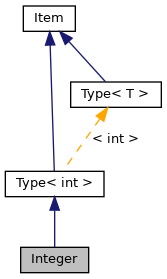
Public Member Functions | |
Integer (const std::string &tag, const std::string &description, int value, Persistent *parent=nullptr) | |
main constructor More... | |
Integer & | operator= (const int &rhs) |
assign from int More... | |
Integer (Integer &&other) | |
move constructor More... | |
Integer & | operator= (Integer &&other) |
move assignment More... | |
const std::string & | type () const override |
void | increment (unsigned int i=1) |
increase by i the value of the Integer; i must be positive More... | |
void | decrement (unsigned int i=1) |
decrease by i the value of the Integer; i must be positive More... | |
![]() | |
Type (const std::string &tag, const std::string &description, Persistent *parent, const int &value) | |
main constructor More... | |
Type (Type &&other) | |
move constructor More... | |
Type & | operator= (Type &&other) |
move assignment More... | |
int & | value (void) |
Type value getter. More... | |
const int & | value (void) const |
bool | operator!= (const Type &rhs) const |
bool | operator== (const Type &rhs) const |
![]() | |
Item (const Item &other) | |
copy constructor copies all elements but parent which is set to nullptr More... | |
Item & | operator= (const Item &other) |
copy assignment operator copies all elements but parent which is set to nullptr More... | |
Item (const std::string &tag, const std::string &description, Persistent *parent) | |
Item (Item &&other) | |
move constructor More... | |
Item & | operator= (Item &&other) |
move assignment operator More... | |
virtual | ~Item () |
const std::string & | tag () const |
const std::string & | description () const |
std::string | fullTag () const |
const Persistent * | parent () const |
virtual const std::string & | type () const =0 |
void | setTag (const std::string &tag) |
void | setDescription (const std::string &description) |
void | setWideTag (const std::wstring &tag) |
wide-character variant, More... | |
void | setWideDescription (const std::wstring &description) |
wide-character variant, More... | |
bool | operator!= (const Item &rhs) const |
bool | operator== (const Item &rhs) const |
Additional Inherited Members | |
![]() | |
typedef int | ValueType |
name the value type to make it possible for function templates acting on Type<T> to access T properties and methods More... | |
![]() | |
int | value_ |
![]() | |
Persistent * | parent_ |
pointer to the parent Persistent; set to this by the constructor if Item is self-standing or a root Persistent of a tree More... | |
Detailed Description
integer variable
used for configuration parameters (nStreams), error numbers (nErrors, nWarnings) etc.
#include <libpf/persistency/Integer.h>
- Remarks
- Warning
- Thread safe:
- Extendable:
- Platform dependencies:
Constructor & Destructor Documentation
◆ Integer() [1/2]
|
inline |
main constructor
◆ Integer() [2/2]
|
inline |
move constructor
Member Function Documentation
◆ decrement()
void Integer::decrement | ( | unsigned int | i = 1 | ) |
decrease by i the value of the Integer; i must be positive
◆ increment()
void Integer::increment | ( | unsigned int | i = 1 | ) |
increase by i the value of the Integer; i must be positive
◆ operator=() [1/2]
Integer & Integer::operator= | ( | const int & | rhs | ) |
assign from int
◆ operator=() [2/2]
◆ type()
|
overridevirtual |
The documentation for this class was generated from the following file: