Semi-abstract class, with a type, a tag and a description; also knows about its parent so can be part of a hierarchical data structure. More...
#include <Item.h>
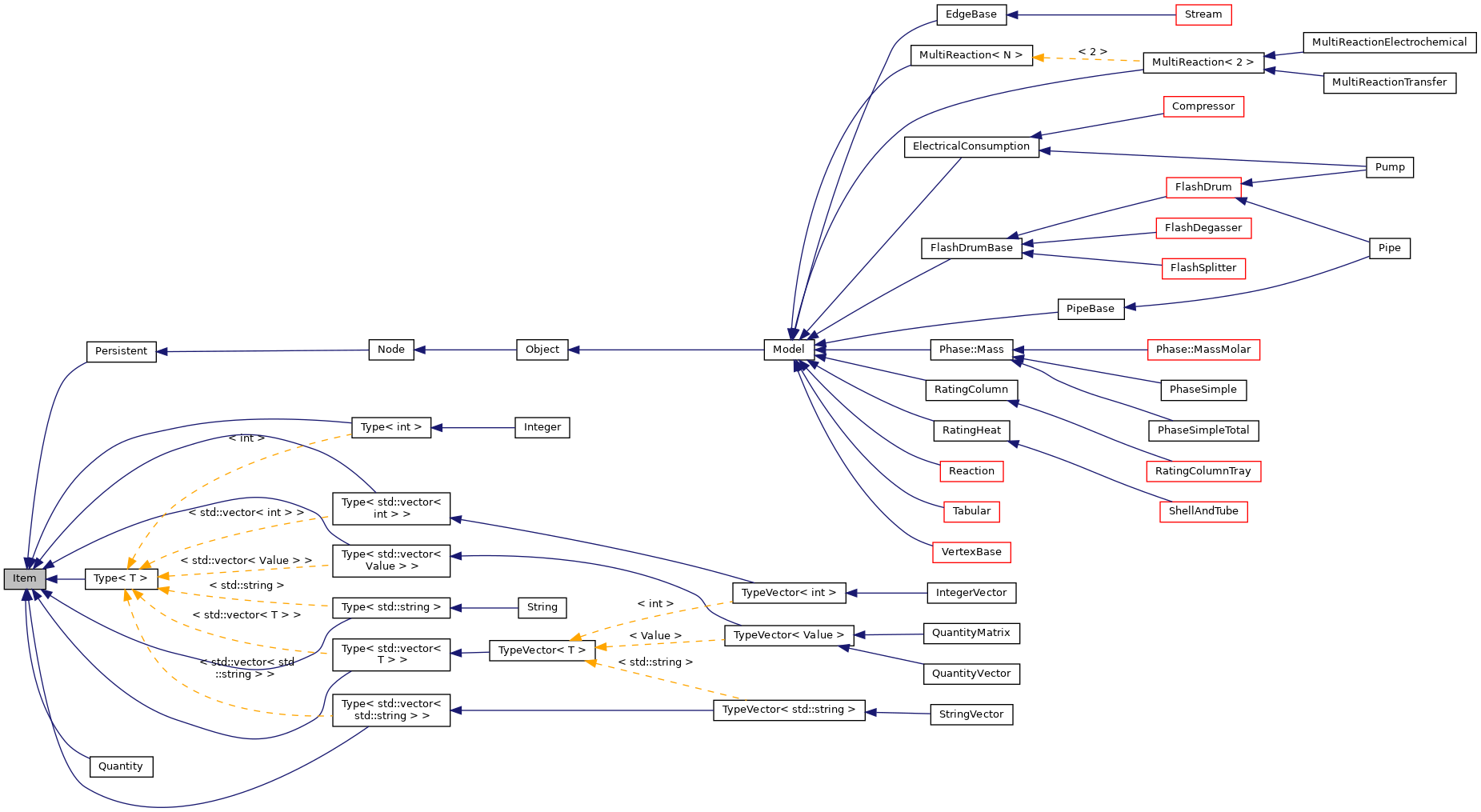
Public Member Functions | |
Item (const Item &other) | |
copy constructor copies all elements but parent which is set to nullptr More... | |
Item & | operator= (const Item &other) |
copy assignment operator copies all elements but parent which is set to nullptr More... | |
Item (const std::string &tag, const std::string &description, Persistent *parent) | |
Item (Item &&other) | |
move constructor More... | |
Item & | operator= (Item &&other) |
move assignment operator More... | |
virtual | ~Item () |
const std::string & | tag () const |
const std::string & | description () const |
std::string | fullTag () const |
const Persistent * | parent () const |
virtual const std::string & | type () const =0 |
void | setTag (const std::string &tag) |
void | setDescription (const std::string &description) |
void | setWideTag (const std::wstring &tag) |
wide-character variant, More... | |
void | setWideDescription (const std::wstring &description) |
wide-character variant, More... | |
bool | operator!= (const Item &rhs) const |
bool | operator== (const Item &rhs) const |
Protected Attributes | |
Persistent * | parent_ |
pointer to the parent Persistent; set to this by the constructor if Item is self-standing or a root Persistent of a tree More... | |
Friends | |
class | Node |
Detailed Description
Semi-abstract class, with a type, a tag and a description; also knows about its parent so can be part of a hierarchical data structure.
#include <libpf/persistency/Item.h>
- Remarks
Constructor & Destructor Documentation
◆ Item() [1/3]
Item::Item | ( | const Item & | other | ) |
copy constructor copies all elements but parent which is set to nullptr
◆ Item() [2/3]
Item::Item | ( | const std::string & | tag, |
const std::string & | description, | ||
Persistent * | parent | ||
) |
For the admissible characters,
- See also
- setTag and setDescription
- Parameters
-
tag UTF-8 encoded human-readable label, unique among siblings description UTF-8 encoded description parent pointer to the parent Persistent; set to this by the constructor if Item is self-standing or a root Persistent of a tree
- Exceptions
-
ErrorInvalidCharacter if invalid characters are present in tag or in description
◆ Item() [3/3]
Item::Item | ( | Item && | other | ) |
move constructor
◆ ~Item()
|
inlinevirtual |
Member Function Documentation
◆ description()
const std::string & Item::description | ( | ) | const |
- Returns
- the UTF-8 encoded description
- Note
- it is the responsibility of the caller to not access this reference after the lifecycle of the Item
◆ fullTag()
std::string Item::fullTag | ( | ) | const |
- Returns
- the UTF-8 encoded full human-readable label, unique within the scope of the tree
◆ operator!=()
bool Item::operator!= | ( | const Item & | rhs | ) | const |
- Returns
- true if the two Items are different
◆ operator=() [1/2]
copy assignment operator copies all elements but parent which is set to nullptr
◆ operator=() [2/2]
◆ operator==()
bool Item::operator== | ( | const Item & | rhs | ) | const |
- Returns
- true if the two Items are identical
◆ parent()
|
inline |
- Returns
- a pointer to the parent Persistent object
◆ setDescription()
void Item::setDescription | ( | const std::string & | description | ) |
Sets the description, UTF-8 encoded. The first character of the description must be alphabetic, while the subsequent characters may be alphanumeric, space or any of the following characters: ,:-_{}<>[]. For the definition of alphabetic and alphanumeric characters,
- See also
- setTag
- Parameters
-
description UTF-8 encoded descriptive text
- Exceptions
-
ErrorInvalidCharacter if invalid characters are present
◆ setTag()
void Item::setTag | ( | const std::string & | tag | ) |
Sets the tag, a UTF-8 encoded human-readable label, unique among siblings. The first character of the tag must be alphabetic, while the subsequent characters may be alphanumeric, space or any of the following characters: ,-_{}<> thus compared to the description, the :[] characters are specifically excluded. By alphabetic character the characters in the a - z, A - Z range are intended (case sensitive), along with all the accented characters and generally all the characters considered alphabetic in the main languages. By alphanumeric character an alphabetic character or a digit (0 - 9) is intended.
- Parameters
-
tag human-readable UTF-8 encoded label, for a Node belonging to a tree must be unique among siblings
- Exceptions
-
ErrorInvalidCharacter if invalid characters are present
◆ setWideDescription()
void Item::setWideDescription | ( | const std::wstring & | description | ) |
wide-character variant,
- See also
- setDescription
◆ setWideTag()
void Item::setWideTag | ( | const std::wstring & | tag | ) |
wide-character variant,
- See also
- setTag
◆ tag()
const std::string & Item::tag | ( | ) | const |
- Returns
- the UTF-8 encoded human-readable label, unique among siblings
- Note
- it is the responsibility of the caller to not access this reference after the lifecycle of the Item
◆ type()
|
pure virtual |
- Returns
- the C++ name of the class the instance belongs to
- Note
- it is the responsibility of the caller to not access this reference after the lifecycle of the Item
Implemented in Integer, IntegerVector, Quantity, QuantityMatrix, QuantityVector, String, StringVector, Column, ColumnSection, Decanter, FallingFilm, LiquidRingVacuumPump, CompressionStage, MultiCompressorIntercooled< N >, MultiStage, MultiStage2D, MultiStageOneTwo, PressureSwingAbsorption, RatingColumnTraySieve, ReboilerCrossFlow, Terminator, Zone, Node, Object, PhaseActivity< U >, PhaseGeneric< T >, PhaseGeneric< U >, PhaseSimple, PhaseSimpleTotal, PhaseTotal, StreamIapwsLiquidVapor, StreamOne, StreamOneSolid, StreamSimple< NV, NL, NS >, StreamTabularLiquidLiquid, StreamTabularLiquidVapor, StreamTwo< F >, StreamTwo< FlashVl >, StreamTwoSolid< F >, Compressor, Degasser, Divider, Ejector, Exchanger, FlashDegasser, FlashDrum, FlashSplitter, HeatTransfer, HtuNtu, Mixer, MultiExchanger, Multiplier, MultiReaction< N >, MultiReaction< 2 >, MultiReactionElectrochemical, MultiReactionTransfer, Pipe, Pump, RatingHeat, RatingHeatShellAndTubeFallingFilmReboiler, RatingHeatShellAndTubeHeater, RatingHeatShellAndTubeRecovery, RatingHeatShellAndTubeThermosiphon, Reaction, ReactionEquilibrium, ReactionOxidationHydrocarbon, ReactionWaterGasShift, ReactionWaterGasShiftEquilibrium, ReactionOxidationEquilibriumCH4, ReactionOxidationCO, ReactionWaterGasC, ReactionReformingCH4, ReactionReformingEquilibriumCH4, ReactionReformingC2H6, ReactionReformingEquilibriumC2H6, ReactionReformingC3H8, ReactionReformingEquilibriumC3H8, ReactionOxidationMeOH, ReactionReformingMeOH, ReactionReformingEquilibriumMeOH, ReactionSynthesisNH3, ReactionSynthesisEquilibriumNH3, ReactionOxidationN2, ReactionOxidationEquilibriumN2, ReactionOxidationC2H6, ReactionOxidationNH3, ReactionOxidationPhenol, ReactionYield, Selector, Separator, ShellAndTube, and Splitter.
Friends And Related Function Documentation
◆ Node
|
friend |
Member Data Documentation
◆ parent_
|
protected |
pointer to the parent Persistent; set to this by the constructor if Item is self-standing or a root Persistent of a tree
The documentation for this class was generated from the following file: