#include <FlashBase.h>
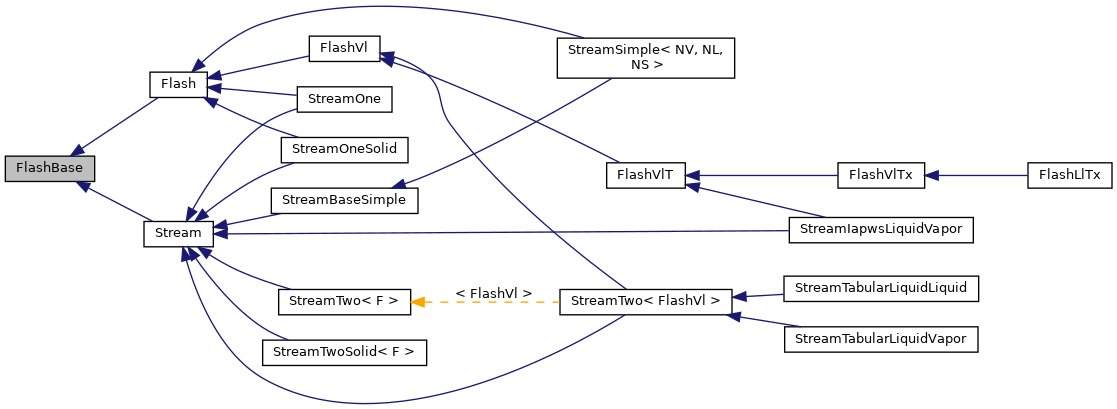
Public Member Functions | |
FlashBase (void) | |
void | setIdeal (bool i) |
bool | isIdeal (void) |
virtual SolverNleInterface * | solver (void)=0 |
virtual int | niter (void) const =0 |
return Flash iterations More... | |
virtual void | estimate (const Value &Pres, const Value &Temp)=0 |
const Value & | Temperature (void) const |
const Value & | Pressure (void) const |
virtual const Value & | Fraction (Libpf::Utility::PhaseIndex p, bool clip=false) const =0 |
during Flash iterations use this function to get the actual estimate of the fraction of phase p More... | |
virtual Vector | X (Libpf::Utility::PhaseIndex p) const =0 |
virtual const Value & | Sumx (Libpf::Utility::PhaseIndex p) const =0 |
virtual bool | converged (void) const =0 |
returns true if the flash has been solved successfully, More... | |
Protected Member Functions | |
virtual | ~FlashBase ()=default |
virtual const Value & | calculateTotalP (const Value &rho) const =0 |
virtual const Value & | calculateTotalRho (void) const =0 |
calculates total molar density for all phases in kmol/m3 More... | |
virtual const Value & | calculateTotalH (void) const =0 |
calculates molar enthalpy for all phases in J/kmol based on current values of the hidden unknowns More... | |
virtual const Value & | calculateTotalS (void) const =0 |
calculates molar entropy for all phases in J/kmol/K based on current values of the hidden unknowns More... | |
virtual void | prepare_residuals (void)=0 |
virtual void | prepare_Flash_residuals (void)=0 |
virtual int | calculateResidualsEos (std::vector< Value > &y, uint32_t offset)=0 |
virtual void | estimateEos (const Value &Pres, const Value &Temp)=0 |
estimate eos roots-related unknowns More... | |
virtual int | calculateResidualsEquilibrium (std::vector< Value > &y, uint32_t offset)=0 |
virtual void | estimateEquilibrium (const Value &Pres, const Value &Temp)=0 |
estimate equilibrium-related unknowns More... | |
virtual void | setFlash_ (const Libpf::Utility::FlashMode &fm, const Value &x, const Value &y, bool strict=true)=0 |
Set the stream's state variables and the Flash. More... | |
virtual int | solveFlash (bool simultaneous, bool skip)=0 |
virtual std::string | message (int code)=0 |
virtual void | setup_ (std::vector< Value * > pbeta_, std::vector< const double * > pbetamin_, std::vector< const double * > pbetamax_)=0 |
Protected Attributes | |
Libpf::Utility::FlashMode | fm_ |
bool | zero_residuals_ |
used to skip Flash residuals calculations if stream is empty More... | |
Value | T_ |
Temperature, unknown. More... | |
Value | P_ |
Pressure, unknown. More... | |
Value | Pset_ |
specified pressure, Pa More... | |
Value | Tset_ |
specified temperature, K More... | |
Value | Hset_ |
specified molar enthalpy, J/kmol More... | |
Value | Sset_ |
specified molar entropy, J/(kmol*K) More... | |
Value | rhoset_ |
specified molar density, kmol/m3 More... | |
Detailed Description
Common stuff to all Flashes including single phase Flashes
#include <libpf/streams/Flash.h>
- Remarks
- Warning
- Thread safe:
- Extendable:
- Platform dependencies:
Constructor & Destructor Documentation
◆ ~FlashBase()
|
protectedvirtualdefault |
◆ FlashBase()
FlashBase::FlashBase | ( | void | ) |
Member Function Documentation
◆ calculateResidualsEos()
|
protectedpure virtual |
fill in residuals for the calculation of {vapor, liquid} eos roots as required returns the number of eos roots that are computed
Implemented in Stream.
◆ calculateResidualsEquilibrium()
|
protectedpure virtual |
fill in residuals for the calculation phase equilibria as required returns the number of equations that are computed
Implemented in StreamOne, StreamOneSolid, StreamSimple< NV, NL, NS >, StreamIapwsLiquidVapor, StreamTwo< F >, StreamTwo< FlashVl >, and StreamTwoSolid< F >.
◆ calculateTotalH()
|
protectedpure virtual |
calculates molar enthalpy for all phases in J/kmol based on current values of the hidden unknowns
Implemented in Stream.
◆ calculateTotalP()
◆ calculateTotalRho()
|
protectedpure virtual |
calculates total molar density for all phases in kmol/m3
Implemented in Stream.
◆ calculateTotalS()
|
protectedpure virtual |
calculates molar entropy for all phases in J/kmol/K based on current values of the hidden unknowns
Implemented in Stream.
◆ converged()
|
pure virtual |
returns true if the flash has been solved successfully,
- See also
- SolverNleInterface::nonConverged
Implemented in Flash.
◆ estimate()
◆ estimateEos()
|
protectedpure virtual |
estimate eos roots-related unknowns
Implemented in Stream.
◆ estimateEquilibrium()
|
protectedpure virtual |
estimate equilibrium-related unknowns
Implemented in Flash, StreamIapwsLiquidVapor, StreamTwo< F >, StreamTwo< FlashVl >, and StreamTwoSolid< F >.
◆ Fraction()
|
pure virtual |
during Flash iterations use this function to get the actual estimate of the fraction of phase p
- Parameters
-
p index of the phase clip whether to clip the fraction between 0 and 1
Implemented in Flash, FlashVl, StreamOneSolid, and StreamTwoSolid< F >.
◆ isIdeal()
bool FlashBase::isIdeal | ( | void | ) |
◆ message()
|
protectedpure virtual |
Implemented in Flash.
◆ niter()
|
pure virtual |
◆ prepare_Flash_residuals()
|
protectedpure virtual |
◆ prepare_residuals()
|
protectedpure virtual |
to be called before evaluating the residuals; implement in stream or its subclasses; put any Flash-specific initialization stuff here
Implemented in Stream, StreamOne, StreamOneSolid, StreamTwo< F >, StreamTwo< FlashVl >, and StreamTwoSolid< F >.
◆ Pressure()
const Value & FlashBase::Pressure | ( | void | ) | const |
during Flash iterations use this function to get the actual estimate of the pressure
◆ setFlash_()
|
protectedpure virtual |
◆ setIdeal()
void FlashBase::setIdeal | ( | bool | i | ) |
Setter for the ideal flash calculation
- Parameters
-
i true = ideal calculation false = not-ideal calculation
◆ setup_()
|
protectedpure virtual |
◆ solveFlash()
|
protectedpure virtual |
solve the flash if !simultaneous, only calls calculateResiduals if simultaneous
- Parameters
-
simultaneous used if the flash is to solved during a simultaneous resolution of a larger set of equations skip skip flash calculation and force the residuals to zero; used for streams with negligible flow
Implemented in Flash.
◆ solver()
|
pure virtual |
Implemented in Flash.
◆ Sumx()
|
pure virtual |
◆ Temperature()
const Value & FlashBase::Temperature | ( | void | ) | const |
during Flash iterations use this function to get the actual estimate of the temperature
◆ X()
|
pure virtual |
during Flash iterations use this function to get the actual estimate of the molar fractions for phase p
Implemented in Flash, FlashVl, StreamOneSolid, and StreamTwoSolid< F >.
Member Data Documentation
◆ fm_
|
protected |
used to store the current Flash specification; can be different from the FlashBasemode specified in the stream ( FlashBaseoption ) i.e. if PT stream is connected as outlet of a compressor it will be PS FlashBaseed
◆ Hset_
|
protected |
specified molar enthalpy, J/kmol
◆ P_
|
protected |
Pressure, unknown.
◆ Pset_
|
protected |
specified pressure, Pa
◆ rhoset_
|
protected |
specified molar density, kmol/m3
◆ Sset_
|
protected |
specified molar entropy, J/(kmol*K)
◆ T_
|
protected |
Temperature, unknown.
◆ Tset_
|
protected |
specified temperature, K
◆ zero_residuals_
|
protected |
used to skip Flash residuals calculations if stream is empty
The documentation for this class was generated from the following file: