differentiate calculateResiduals and calculateResidualsLocal More...
#include <ObjectiveNleAd.h>
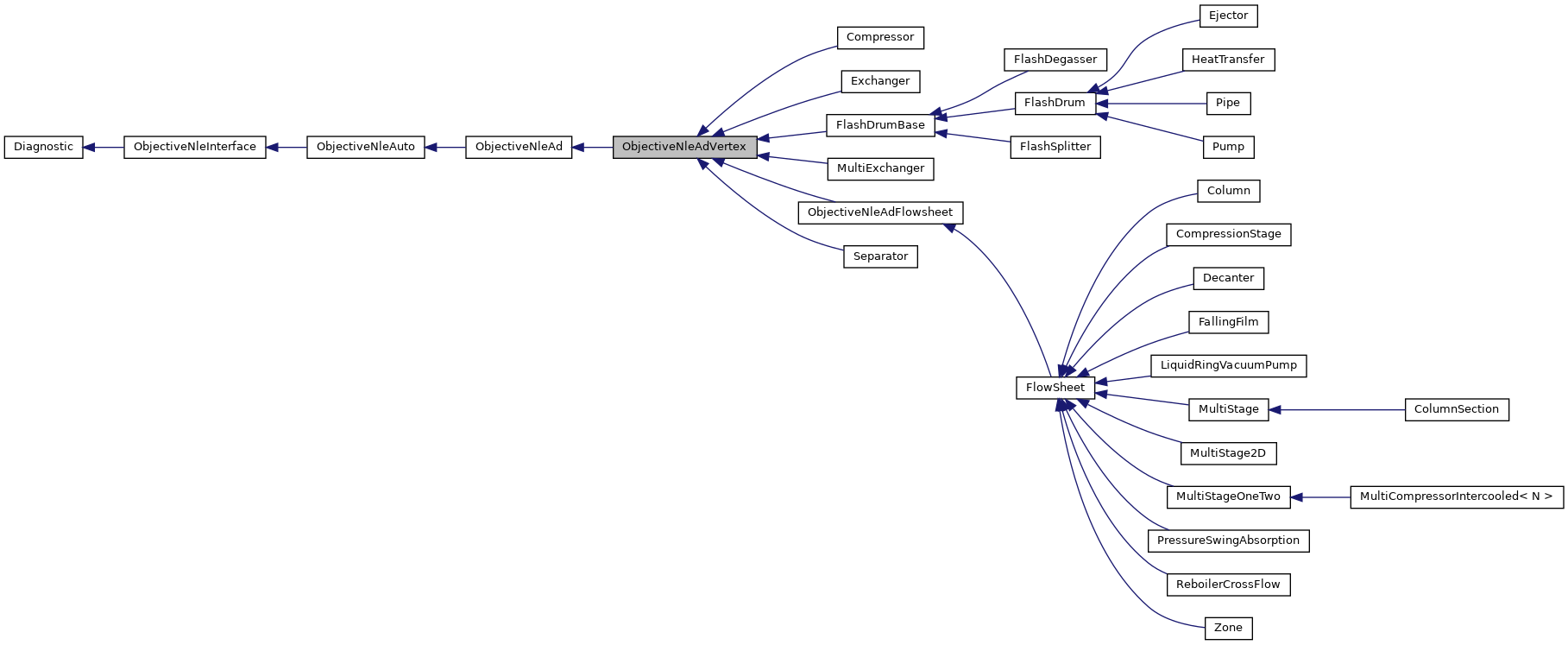
Public Member Functions | |
ObjectiveNleAdVertex (std::string t) | |
constructor More... | |
void | unscalex (double *x) override |
transforms back the vector of scaled variables x by applying the unscaling More... | |
int | calculateResiduals (std::vector< Value > &y, uint32_t offset) override |
void | operator() (double *, double *, Jacobian *) override |
implements the () operator by calling calculateResidualsLocal and calculateResiduals; overrides ObjectiveNleAd::operator() More... | |
virtual int | calculateResidualsLocal (void)=0 |
compute residuals and store into local vector y_; must be provided by implementations More... | |
virtual void | setup_of (void)=0 |
setup and resize appropriately internal vectors; must be provided by implementations More... | |
![]() | |
ObjectiveNleAd (std::string t) | |
constructor More... | |
double | xmin (unsigned int i) |
return the lower bound on unknown i More... | |
double | xmax (unsigned int i) |
return the upper bound on unknown i More... | |
unsigned int | size (void) const override |
returns the current size of the problem More... | |
void | scalex (double *x) override |
transforms the vector of "real" variables x by applying the scaling More... | |
void | unscalex (double *x) override |
transforms back the vector of scaled variables x by applying the unscaling More... | |
void | operator() (double *, double *, Jacobian *J) override |
implements the () operator by calling calculateResiduals; derived class may override it More... | |
void | addUnknown (Value *x, Scaler scaler, const std::string &tag) |
void | changeUnknown (unsigned int i, Value *x, Scaler scaler, const std::string &tag) |
change the i-th unknown More... | |
void | addObjectiveFunction (ObjectiveNleAd &of) |
append the remote objective function More... | |
![]() | |
ObjectiveNleAuto (std::string t) | |
constructor More... | |
virtual unsigned int | size (void) const |
returns the current size of the problem More... | |
bool | init (double *x) |
void | setValue (unsigned int i, double x) |
copies the value for the i-th unknown from x in the Value pointed to, without altering the derivatives More... | |
void | copyresults (double *x) |
copies the unknown values from the x vector into the Values pointed to More... | |
void | inactivate (void) |
inactivates all unknowns More... | |
void | clearderivatives (void) |
clears the derivative information for all unknowns without setting to inactive More... | |
void | setActive (unsigned int i) |
activates the i-th unknown More... | |
void | scalex (double *) override |
transforms the vector of "real" variables x by applying the scaling More... | |
void | unscalex (double *x) override |
transforms back the vector of scaled variables x by applying the unscaling More... | |
void | operator() (double *, double *, Jacobian *J) override |
implements the () operator by calling calculateResiduals; derived class may override it More... | |
void | setx (unsigned int i, Value &x) |
sets the i-th unknown to x More... | |
const Value & | unknown (unsigned int i) const |
returns a reference to the i-th unknown More... | |
const std::string & | unknownTag (unsigned int i) const |
returns a reference to the tag of the i-th unknown More... | |
double | xmin (unsigned int i) |
return the lower bound on unknown i More... | |
double | xmax (unsigned int i) |
return the upper bound on unknown i More... | |
void | print (void) const |
lists unknowns More... | |
const std::string & | tagof (void) const |
returns the tag of the instance More... | |
void | settagof (std::string t) |
sets the tag of the instance More... | |
void | appendtagof (const std::string &t) |
appends to the tag of the instance More... | |
int | zero_residuals (std::vector< Value > &y, uint32_t offset) |
virtual int | calculateResiduals (std::vector< Value > &y, uint32_t offset)=0 |
void | addUnknown (Value *x, const std::string &tag) |
void | changeUnknown (unsigned int i, Value *x, const std::string &tag) |
change the i-th unknown More... | |
![]() | |
ObjectiveNleInterface (void) | |
virtual void | operator() (double *x, double *y, Jacobian *J)=0 |
computes the objective function vector y at the point x and optionally the Jacobian J More... | |
virtual void | scalex (double *x)=0 |
transforms the vector of "real" variables x by applying the scaling More... | |
virtual void | unscalex (double *x)=0 |
transforms back the vector of scaled variables x by applying the unscaling More... | |
![]() | |
Diagnostic (void) | |
void | setVerbosity (int verbosity) |
sets the verbosity level for this instance More... | |
int | verbosity (void) const |
Protected Attributes | |
bool | set_ |
true if setup_of has already been called More... | |
![]() | |
std::vector< Scaler > | scaler_ |
provide scaling and unscaling More... | |
![]() | |
std::vector< Value > | residuals |
Residuals. More... | |
![]() | |
int | verbosityInstance |
Additional Inherited Members | |
![]() | |
int | verbosityLocal |
![]() | |
void | prepare_ (double *x, Jacobian *J) override |
uscales x (unknowns) values from calling function and puts them in x_ Quantities More... | |
void | packResults_ (double *x, double *y, Jacobian *J) |
~ObjectiveNleAd () | |
![]() | |
~ObjectiveNleAuto () | |
![]() | |
~ObjectiveNleInterface () | |
![]() | |
virtual | ~Diagnostic ()=default |
Detailed Description
differentiate calculateResiduals and calculateResidualsLocal
#include <libpf/core/ObjectiveNleAd.h>
- Remarks
- Warning
- Thread safe:
- Extendable:
- Platform dependencies:
Constructor & Destructor Documentation
◆ ObjectiveNleAdVertex()
|
inline |
constructor
Member Function Documentation
◆ calculateResiduals()
|
overridevirtual |
evaluates the residuals, placing them into the supplied vector at the given offset
- Parameters
-
[out] y the vector of residuals to fill in [in] offset the offset inside the vector of residuals
- Returns
- the number of evaluated residuals
- Note
- by contract, implementations should write some value in the y[offset] .. y[offset+returned:value-1] and leave the rest of the vector unchanged
- if the objective function is designed to be solved by itself (i.e. not part of an assembly of objective functions, the offset parameter can be safely ignored
Implements ObjectiveNleAuto.
◆ calculateResidualsLocal()
|
pure virtual |
compute residuals and store into local vector y_; must be provided by implementations
Implemented in FlowSheet, Compressor, Exchanger, FlashDrumBase, MultiExchanger, and Separator.
◆ operator()()
|
overridevirtual |
implements the () operator by calling calculateResidualsLocal and calculateResiduals; overrides ObjectiveNleAd::operator()
Reimplemented from ObjectiveNleAd.
◆ setup_of()
|
pure virtual |
setup and resize appropriately internal vectors; must be provided by implementations
Implemented in FlowSheet, Compressor, Exchanger, FlashDegasser, FlashDrum, FlashDrumBase, FlashSplitter, MultiExchanger, and Separator.
◆ unscalex()
|
overridevirtual |
transforms back the vector of scaled variables x by applying the unscaling
Reimplemented from ObjectiveNleAd.
Member Data Documentation
◆ set_
|
protected |
true if setup_of has already been called
The documentation for this class was generated from the following file: