objective function with analytical derivatives computed with FADOO (Forward Automatic Differentiation with Operator Overloading) More...
#include <ObjectiveNleAuto.h>
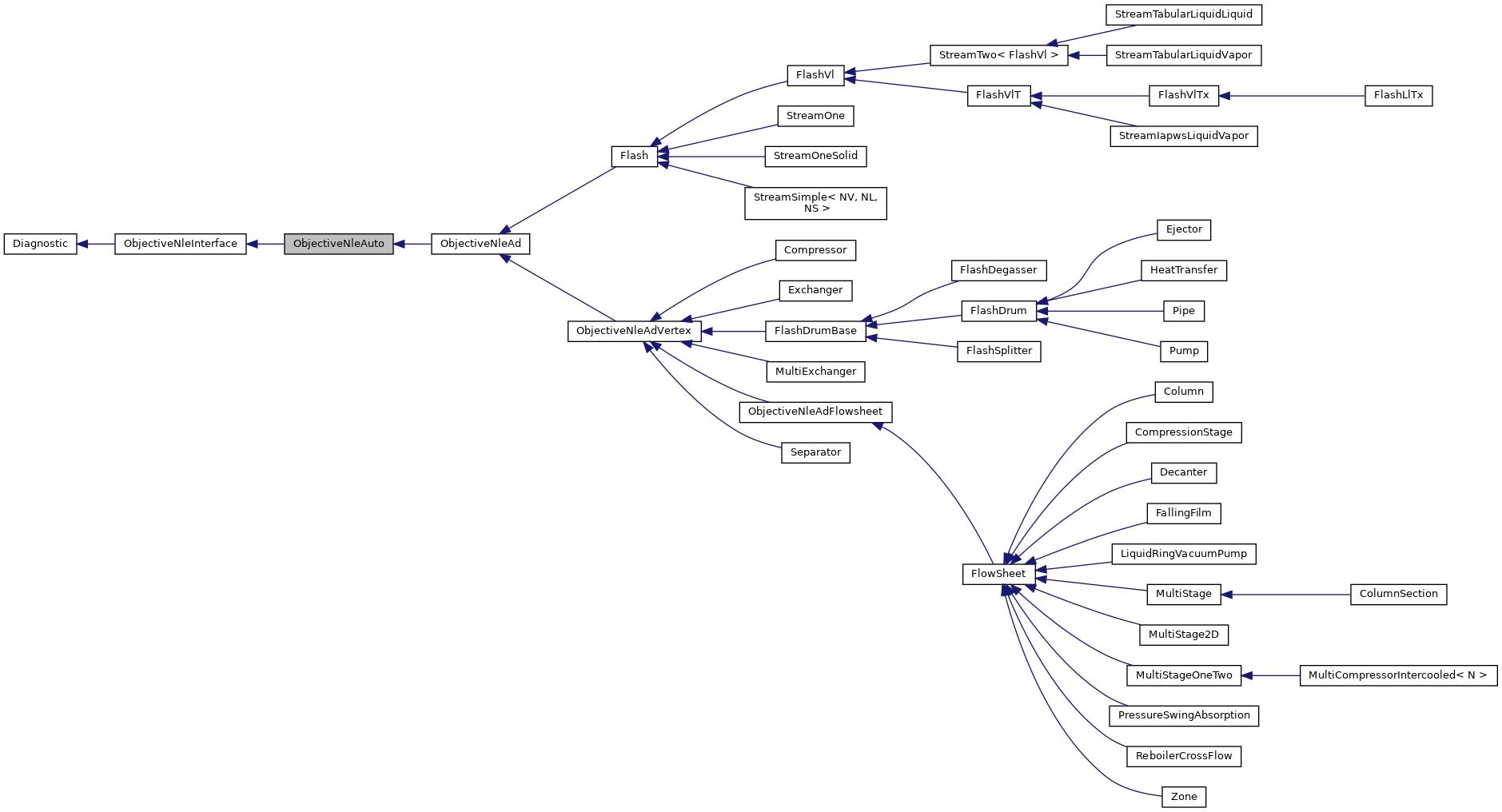
Public Member Functions | |
ObjectiveNleAuto (std::string t) | |
constructor More... | |
virtual unsigned int | size (void) const |
returns the current size of the problem More... | |
bool | init (double *x) |
void | setValue (unsigned int i, double x) |
copies the value for the i-th unknown from x in the Value pointed to, without altering the derivatives More... | |
void | copyresults (double *x) |
copies the unknown values from the x vector into the Values pointed to More... | |
void | inactivate (void) |
inactivates all unknowns More... | |
void | clearderivatives (void) |
clears the derivative information for all unknowns without setting to inactive More... | |
void | setActive (unsigned int i) |
activates the i-th unknown More... | |
void | scalex (double *) override |
transforms the vector of "real" variables x by applying the scaling More... | |
void | unscalex (double *x) override |
transforms back the vector of scaled variables x by applying the unscaling More... | |
void | operator() (double *, double *, Jacobian *J) override |
implements the () operator by calling calculateResiduals; derived class may override it More... | |
void | setx (unsigned int i, Value &x) |
sets the i-th unknown to x More... | |
const Value & | unknown (unsigned int i) const |
returns a reference to the i-th unknown More... | |
const std::string & | unknownTag (unsigned int i) const |
returns a reference to the tag of the i-th unknown More... | |
double | xmin (unsigned int i) |
return the lower bound on unknown i More... | |
double | xmax (unsigned int i) |
return the upper bound on unknown i More... | |
void | print (void) const |
lists unknowns More... | |
const std::string & | tagof (void) const |
returns the tag of the instance More... | |
void | settagof (std::string t) |
sets the tag of the instance More... | |
void | appendtagof (const std::string &t) |
appends to the tag of the instance More... | |
int | zero_residuals (std::vector< Value > &y, uint32_t offset) |
virtual int | calculateResiduals (std::vector< Value > &y, uint32_t offset)=0 |
void | addUnknown (Value *x, const std::string &tag) |
void | changeUnknown (unsigned int i, Value *x, const std::string &tag) |
change the i-th unknown More... | |
![]() | |
ObjectiveNleInterface (void) | |
virtual void | operator() (double *x, double *y, Jacobian *J)=0 |
computes the objective function vector y at the point x and optionally the Jacobian J More... | |
virtual void | scalex (double *x)=0 |
transforms the vector of "real" variables x by applying the scaling More... | |
virtual void | unscalex (double *x)=0 |
transforms back the vector of scaled variables x by applying the unscaling More... | |
![]() | |
Diagnostic (void) | |
void | setVerbosity (int verbosity) |
sets the verbosity level for this instance More... | |
int | verbosity (void) const |
Protected Member Functions | |
~ObjectiveNleAuto () | |
![]() | |
~ObjectiveNleInterface () | |
![]() | |
virtual | ~Diagnostic ()=default |
Protected Attributes | |
std::vector< Value > | residuals |
Residuals. More... | |
![]() | |
int | verbosityInstance |
Friends | |
class | SolverNleInterface |
class | ObjectiveNleAd |
Additional Inherited Members | |
![]() | |
int | verbosityLocal |
Detailed Description
objective function with analytical derivatives computed with FADOO (Forward Automatic Differentiation with Operator Overloading)
Constructor & Destructor Documentation
◆ ~ObjectiveNleAuto()
|
inlineprotected |
◆ ObjectiveNleAuto()
ObjectiveNleAuto::ObjectiveNleAuto | ( | std::string | t | ) |
constructor
Member Function Documentation
◆ addUnknown()
void ObjectiveNleAuto::addUnknown | ( | Value * | x, |
const std::string & | tag | ||
) |
append unknown x with tag to the list of unknowns
- Returns
- the index of the new unknown
◆ appendtagof()
void ObjectiveNleAuto::appendtagof | ( | const std::string & | t | ) |
appends to the tag of the instance
◆ calculateResiduals()
|
pure virtual |
evaluates the residuals, placing them into the supplied vector at the given offset
- Parameters
-
[out] y the vector of residuals to fill in [in] offset the offset inside the vector of residuals
- Returns
- the number of evaluated residuals
- Note
- by contract, implementations should write some value in the y[offset] .. y[offset+returned:value-1] and leave the rest of the vector unchanged
- if the objective function is designed to be solved by itself (i.e. not part of an assembly of objective functions, the offset parameter can be safely ignored
Implemented in ObjectiveNleAdVertex, and Flash.
◆ changeUnknown()
void ObjectiveNleAuto::changeUnknown | ( | unsigned int | i, |
Value * | x, | ||
const std::string & | tag | ||
) |
change the i-th unknown
◆ clearderivatives()
void ObjectiveNleAuto::clearderivatives | ( | void | ) |
clears the derivative information for all unknowns without setting to inactive
◆ copyresults()
void ObjectiveNleAuto::copyresults | ( | double * | x | ) |
copies the unknown values from the x vector into the Values pointed to
◆ inactivate()
void ObjectiveNleAuto::inactivate | ( | void | ) |
inactivates all unknowns
◆ init()
bool ObjectiveNleAuto::init | ( | double * | x | ) |
copies the current unknowns values from the Quantities pointed to by xp_ in the x vector return true if errors are encountered
◆ operator()()
|
overridevirtual |
implements the () operator by calling calculateResiduals; derived class may override it
Implements ObjectiveNleInterface.
◆ print()
void ObjectiveNleAuto::print | ( | void | ) | const |
lists unknowns
◆ scalex()
|
inlineoverridevirtual |
transforms the vector of "real" variables x by applying the scaling
Implements ObjectiveNleInterface.
◆ setActive()
void ObjectiveNleAuto::setActive | ( | unsigned int | i | ) |
activates the i-th unknown
◆ settagof()
void ObjectiveNleAuto::settagof | ( | std::string | t | ) |
sets the tag of the instance
◆ setValue()
void ObjectiveNleAuto::setValue | ( | unsigned int | i, |
double | x | ||
) |
copies the value for the i-th unknown from x in the Value pointed to, without altering the derivatives
◆ setx()
void ObjectiveNleAuto::setx | ( | unsigned int | i, |
Value & | x | ||
) |
sets the i-th unknown to x
◆ size()
|
virtual |
returns the current size of the problem
Reimplemented in ObjectiveNleAd.
◆ tagof()
const std::string & ObjectiveNleAuto::tagof | ( | void | ) | const |
returns the tag of the instance
◆ unknown()
const Value & ObjectiveNleAuto::unknown | ( | unsigned int | i | ) | const |
returns a reference to the i-th unknown
◆ unknownTag()
const std::string & ObjectiveNleAuto::unknownTag | ( | unsigned int | i | ) | const |
returns a reference to the tag of the i-th unknown
◆ unscalex()
|
overridevirtual |
transforms back the vector of scaled variables x by applying the unscaling
Implements ObjectiveNleInterface.
◆ xmax()
double ObjectiveNleAuto::xmax | ( | unsigned int | i | ) |
return the upper bound on unknown i
◆ xmin()
double ObjectiveNleAuto::xmin | ( | unsigned int | i | ) |
return the lower bound on unknown i
◆ zero_residuals()
int ObjectiveNleAuto::zero_residuals | ( | std::vector< Value > & | y, |
uint32_t | offset | ||
) |
force all residuals to zero, placing them into the supplied vector at the given offset
- Parameters
-
[out] y the vector of residuals to fill in [in] offset the offset inside the vector of residuals
- Returns
- the number of evaluated residuals
Friends And Related Function Documentation
◆ ObjectiveNleAd
|
friend |
◆ SolverNleInterface
|
friend |
Member Data Documentation
◆ residuals
|
protected |
Residuals.
The documentation for this class was generated from the following file: