TypeVector< T > Class Template Reference
a generic vector Item, header-only More...
#include <TypeVector.h>
Inheritance diagram for TypeVector< T >:
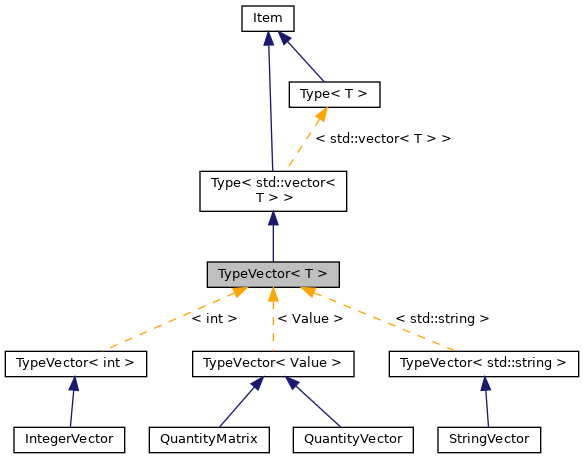
Public Member Functions | |
TypeVector (const std::string &tag, const std::string &description, Persistent *parent, int count, const T &value) | |
main constructor More... | |
TypeVector (TypeVector &&other) | |
move constructor More... | |
TypeVector & | operator= (TypeVector &&other) |
move assignment More... | |
T & | operator[] (std::size_t index) |
const T & | at (std::size_t index) const |
std::size_t | size (void) const |
virtual void | resize (std::size_t count) |
resizes the number of elements contained to count More... | |
void | set (const T &value) |
sets the value for all elements More... | |
![]() | |
Type (const std::string &tag, const std::string &description, Persistent *parent, const std::vector< T > &value) | |
main constructor More... | |
Type (Type &&other) | |
move constructor More... | |
Type & | operator= (Type &&other) |
move assignment More... | |
std::vector< T > & | value (void) |
Type value getter. More... | |
const std::vector< T > & | value (void) const |
bool | operator!= (const Type &rhs) const |
bool | operator== (const Type &rhs) const |
![]() | |
Item (const Item &other) | |
copy constructor copies all elements but parent which is set to nullptr More... | |
Item & | operator= (const Item &other) |
copy assignment operator copies all elements but parent which is set to nullptr More... | |
Item (const std::string &tag, const std::string &description, Persistent *parent) | |
Item (Item &&other) | |
move constructor More... | |
Item & | operator= (Item &&other) |
move assignment operator More... | |
virtual | ~Item () |
const std::string & | tag () const |
const std::string & | description () const |
std::string | fullTag () const |
const Persistent * | parent () const |
virtual const std::string & | type () const =0 |
void | setTag (const std::string &tag) |
void | setDescription (const std::string &description) |
void | setWideTag (const std::wstring &tag) |
wide-character variant, More... | |
void | setWideDescription (const std::wstring &description) |
wide-character variant, More... | |
bool | operator!= (const Item &rhs) const |
bool | operator== (const Item &rhs) const |
Additional Inherited Members | |
![]() | |
typedef std::vector< T > | ValueType |
name the value type to make it possible for function templates acting on Type<T> to access T properties and methods More... | |
![]() | |
std::vector< T > | value_ |
![]() | |
Persistent * | parent_ |
pointer to the parent Persistent; set to this by the constructor if Item is self-standing or a root Persistent of a tree More... | |
Detailed Description
template<class T>
class TypeVector< T >
class TypeVector< T >
a generic vector Item, header-only
#include "TypeVector.h"
- Remarks
- Warning
- Thread safe:
- Extendable:
- Platform dependencies:
Constructor & Destructor Documentation
◆ TypeVector() [1/2]
template<class T >
|
inline |
main constructor
◆ TypeVector() [2/2]
template<class T >
|
inline |
move constructor
Member Function Documentation
◆ at()
template<class T >
const T & TypeVector< T >::at | ( | std::size_t | index | ) | const |
- Returns
- a const reference to the element at the supplied index
- Parameters
-
index index of element to retrieve, 0-base
- Exceptions
-
ErrorRunTime if the index is outside range
◆ operator=()
template<class T >
|
inline |
move assignment
◆ operator[]()
template<class T >
T & TypeVector< T >::operator[] | ( | std::size_t | index | ) |
- Returns
- a reference to the element at the supplied index
- Parameters
-
index index of element to retrieve, 0-base
- Exceptions
-
ErrorRunTime if the index is outside range
◆ resize()
template<class T >
|
inlinevirtual |
resizes the number of elements contained to count
Reimplemented in QuantityMatrix, and QuantityVector.
◆ set()
template<class T >
|
inline |
sets the value for all elements
◆ size()
template<class T >
|
inline |
- Returns
- the number of elements contained
The documentation for this class was generated from the following file: