Concrete class, to represent a persistable node belonging to a hierarchical tree structure; the descendant ids are inside a sequential range of ids [rootId() .. (rootId() + range() - 1)] for a Node belonging to a tree the tag must be unique among siblings; also contains properties and methods related to the visualization (icon, setIcon...) More...
#include <Node.h>
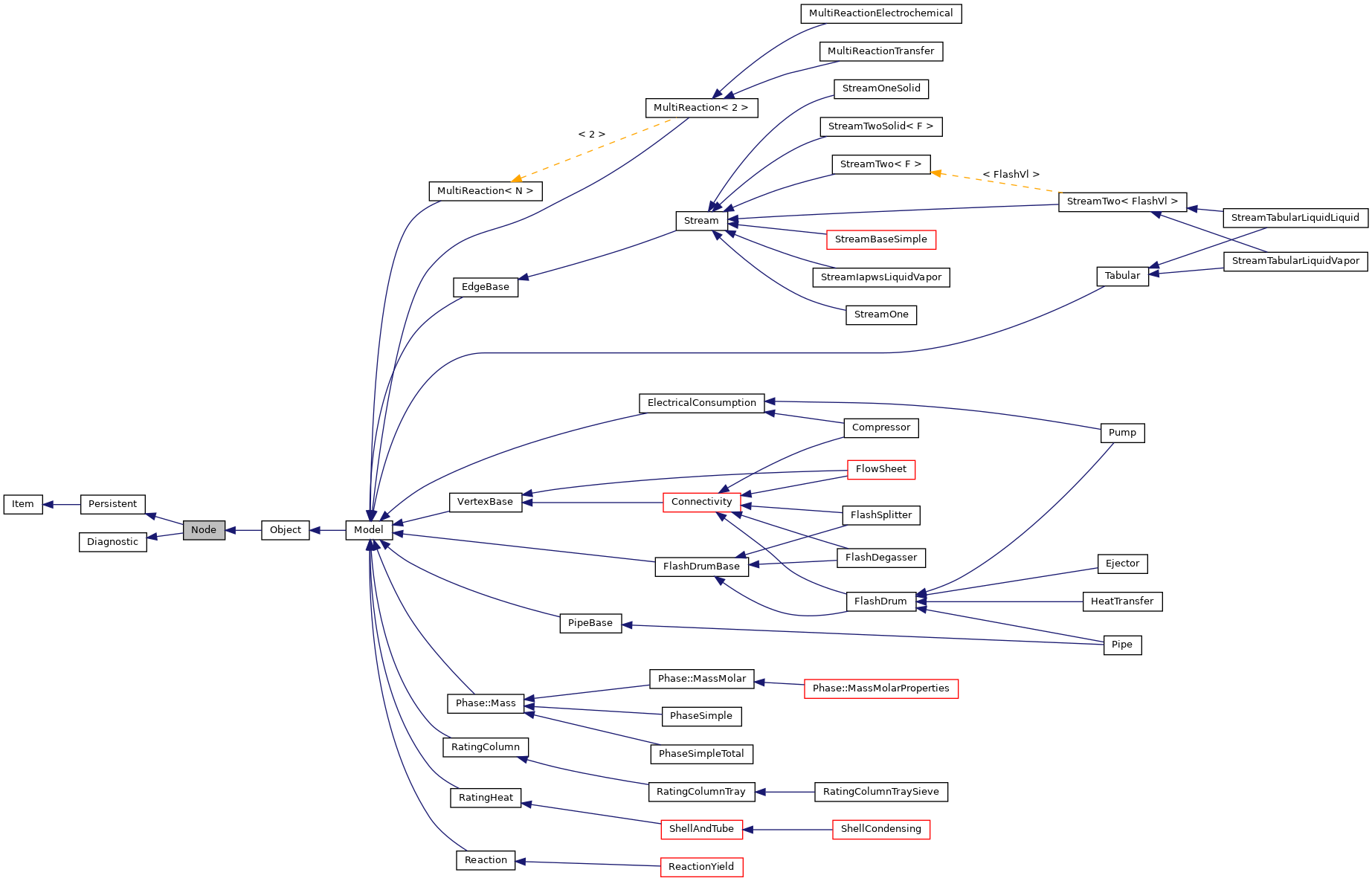
Classes | |
class | ConstIterator |
class | ConstRange |
class | Iterator |
class | Range |
Public Member Functions | |
Node (Libpf::Persistency::Defaults defaults, uint32_t id=0, Persistency *persistency=nullptr, Persistent *parent=nullptr, Persistent *root=nullptr) | |
Node (const Node &other) | |
copy constructor More... | |
Node (Node &&other) | |
move constructor More... | |
virtual std::unique_ptr< Node > | clone (void) const |
virtual Node & | operator= (const Node &other) |
copy assignment operator copies all elements but root which is set to nullptr More... | |
virtual Node & | operator= (Node &&other) |
move assignment More... | |
~Node () | |
int | insert (Persistency *persistency) const override |
int | update (Persistency *persistency) const override |
void | restore (Persistency *persistency) override |
void | remove (Persistency *persistency) const override |
uint32_t | rootId (void) const override |
uint32_t | range (void) const override |
uint32_t | getId (void) override |
returns the next available id and increments the internal counter More... | |
const std::string & | type (void) const override |
virtual std::ostream & | printJson (std::ostream &os, int level=0) const |
print Node in JSON format More... | |
Node & | addChild (std::string type, uint32_t id, Persistency *persistency) |
Node & | addChild (std::string type, Libpf::Persistency::Defaults defaults) |
Node & | addChild (const Node &child) |
Node & | addChild (std::unique_ptr< Node > child) |
std::unique_ptr< Node > | prune (const std::string &tag) |
const Node & | child (const std::string &tag) const |
Node & | at (const std::string &fullRelativeTag) |
const Node & | at (const std::string &fullRelativeTag) const |
uint32_t | descendants (void) const |
Persistent * | root (void) const |
void | renameChild (const std::string &oldName, const std::string &newName) |
bool | existsChild (std::string tag) const |
bool | exists (uint32_t id) |
Node & | search (uint32_t id) |
bool | operator!= (const Node &rhs) const |
bool | operator== (const Node &rhs) const |
virtual void | readVariables (Persistency *) |
reads Q, QV and QM from persistency More... | |
virtual void | readParameters (Persistency *) |
reads I, IV, S and SV from persistency More... | |
bool | isRestored (void) const |
return whether the Node has just been retrieved from persistent storage More... | |
void | setIcon (std::string icon, double width, double height) |
bool | hasIcon (void) const |
std::string | iconName (bool raster) const |
double | iconWidth (void) const |
double | iconHeight (void) const |
const Range | children (void) |
const ConstRange | children (void) const |
![]() | |
Persistent (const std::string &tag, const std::string &description, Persistent *parent, uint32_t id) | |
main constructor More... | |
Persistent (const Persistent &) | |
copy constructor More... | |
Persistent & | operator= (const Persistent &) |
copy assignment More... | |
Persistent (Persistent &&other) | |
move constructor More... | |
Persistent & | operator= (Persistent &&other) |
move assignment More... | |
~Persistent (void) | |
uint32_t | id (void) const |
std::string | uuid (void) const |
uint32_t | parentId (void) const |
virtual uint32_t | rootId (void) const =0 |
virtual uint32_t | range (void) const =0 |
virtual uint32_t | getId (void)=0 |
returns the next available id and increments the internal counter More... | |
double | created_at (void) const |
double | updated_at (void) const |
void | updated_at (double u) const |
virtual int | insert (Persistency *persistency) const =0 |
virtual int | update (Persistency *persistency) const =0 |
virtual void | restore (Persistency *persistency)=0 |
virtual void | remove (Persistency *persistency) const =0 |
![]() | |
Item (const Item &other) | |
copy constructor copies all elements but parent which is set to nullptr More... | |
Item & | operator= (const Item &other) |
copy assignment operator copies all elements but parent which is set to nullptr More... | |
Item (const std::string &tag, const std::string &description, Persistent *parent) | |
Item (Item &&other) | |
move constructor More... | |
Item & | operator= (Item &&other) |
move assignment operator More... | |
virtual | ~Item () |
const std::string & | tag () const |
const std::string & | description () const |
std::string | fullTag () const |
const Persistent * | parent () const |
virtual const std::string & | type () const =0 |
void | setTag (const std::string &tag) |
void | setDescription (const std::string &description) |
void | setWideTag (const std::wstring &tag) |
wide-character variant, More... | |
void | setWideDescription (const std::wstring &description) |
wide-character variant, More... | |
bool | operator!= (const Item &rhs) const |
bool | operator== (const Item &rhs) const |
![]() | |
Diagnostic (void) | |
void | setVerbosity (int verbosity) |
sets the verbosity level for this instance More... | |
int | verbosity (void) const |
Protected Member Functions | |
int | offset (void) const |
![]() | |
virtual | ~Diagnostic ()=default |
Protected Attributes | |
std::map< std::string, std::unique_ptr< Node > > | children_ |
the collection of direct descendants More... | |
![]() | |
Persistent * | parent_ |
pointer to the parent Persistent; set to this by the constructor if Item is self-standing or a root Persistent of a tree More... | |
![]() | |
int | verbosityInstance |
Friends | |
class | Object |
class | Iterator |
std::ostream & | operator<< (std::ostream &os, const Node &node) |
Detailed Description
Concrete class, to represent a persistable node belonging to a hierarchical tree structure; the descendant ids are inside a sequential range of ids [rootId() .. (rootId() + range() - 1)] for a Node belonging to a tree the tag must be unique among siblings; also contains properties and methods related to the visualization (icon, setIcon...)
Constructor & Destructor Documentation
◆ Node() [1/3]
Node::Node | ( | Libpf::Persistency::Defaults | defaults, |
uint32_t | id = 0 , |
||
Persistency * | persistency = nullptr , |
||
Persistent * | parent = nullptr , |
||
Persistent * | root = nullptr |
||
) |
universal constructor; if persistency is not nullptr, restore from database else create a new node
- Parameters
-
defaults default informations to build and configure the Node and its descendants id integer identifier, for a Node belonging to a tree must be unique within the tree; defaults to 0 persistency database connection parent pointer to the parent Node; set to nullptr if Node is self-standing or the root Node in a tree; defaults to nullptr root pointer to the root Node; set to nullptr if Node is self-standing or the root Node in a tree; defaults to nullptr
◆ Node() [2/3]
Node::Node | ( | const Node & | other | ) |
copy constructor
◆ Node() [3/3]
Node::Node | ( | Node && | other | ) |
move constructor
◆ ~Node()
Node::~Node | ( | ) |
Member Function Documentation
◆ addChild() [1/4]
adds a new child copying the supplied Node
- Parameters
-
child the Node to copy
- Returns
- a reference to the newly created Node
- Exceptions
-
if there is already a child with the same label tag
- Note
- for the sequential range guarantee to hold, callers must populate the tree in depth-first order
- it is the responsibility of the caller to not access the returned reference after the lifecycle of the Node is over
◆ addChild() [2/4]
Node & Node::addChild | ( | std::string | type, |
Libpf::Persistency::Defaults | defaults | ||
) |
adds a new child of supplied type, actually creating a new node
- Parameters
-
type type unique identifier defaults default informations to build and configure the Node and its descendants
- Returns
- a reference to the newly created Node
- Exceptions
-
if there is already a child with the same label tag
- Note
- for the sequential range guarantee to hold, callers must populate the tree in depth-first order
- it is the responsibility of the caller to not access the returned reference after the lifecycle of the Node is over
◆ addChild() [3/4]
Node & Node::addChild | ( | std::string | type, |
uint32_t | id, | ||
Persistency * | persistency | ||
) |
adds a new child of supplied type, restoring from database else create a new node
- Parameters
-
type type unique identifier id integer identifier in the database persistency database connection
- Returns
- a reference to the newly created Node
- Exceptions
-
if there is already a child with the same label tag
- Note
- for the sequential range guarantee to hold, callers must populate the tree in depth-first order
- it is the responsibility of the caller to not access the returned reference after the lifecycle of the Node is over
◆ addChild() [4/4]
adds a new child moving the supplied Node
- Parameters
-
child the Node to move
- Returns
- a reference to the newly created Node
- Exceptions
-
if there is already a child with the same label tag
- Note
- for the sequential range guarantee to hold, callers must populate the tree in depth-first order
- it is the responsibility of the caller to not access the returned reference after the lifecycle of the Node is over
◆ at() [1/2]
Node & Node::at | ( | const std::string & | fullRelativeTag | ) |
- Returns
- a reference to the descendant with the supplied full relative label
- Parameters
-
fullRelativeTag the UTF-8 encoded full relative human-readable label, unique within the scope of this Node and all its descendants
- Exceptions
-
if there no descendant with the supplied full relative label tag
- Note
- it is the responsibility of the caller to not access this reference after the lifecycle of the Node is over
◆ at() [2/2]
const Node & Node::at | ( | const std::string & | fullRelativeTag | ) | const |
- Returns
- a const reference to the descendant with the supplied full relative label
- Parameters
-
fullRelativeTag the UTF-8 encoded full relative human-readable label, unique within the scope of this Node and all its descendants
- Exceptions
-
if there no descendant with the supplied full relative label tag
- Note
- it is the responsibility of the caller to not access this reference after the lifecycle of the Node is over
◆ child()
const Node & Node::child | ( | const std::string & | tag | ) | const |
- Returns
- a const reference to the child (direct descendant) with the supplied label tag
- Parameters
-
tag UTF-8 encoded human-readable label
- Exceptions
-
ErrorRunTime if there no child with the supplied label tag
- Note
- it is the responsibility of the caller to not access this reference after the lifecycle of the Node is over
◆ children() [1/2]
const Range Node::children | ( | void | ) |
- Returns
- a Range object that with a range-based for-loop can iterate over all direct descendants
◆ children() [2/2]
const ConstRange Node::children | ( | void | ) | const |
- Returns
- a ConstRange object that with a const range-based for-loop can iterate over all direct descendants
◆ clone()
|
inlinevirtual |
◆ descendants()
uint32_t Node::descendants | ( | void | ) | const |
- Returns
- the total number of descendants, recursively spanning all levels of the tree
◆ exists()
bool Node::exists | ( | uint32_t | id | ) |
- Returns
- true if a descendant with the supplied id exists
◆ existsChild()
bool Node::existsChild | ( | std::string | tag | ) | const |
- Returns
- true if a child with the supplied label exists
- Parameters
-
tag UTF-8 encoded human-readable label
◆ getId()
|
overridevirtual |
returns the next available id and increments the internal counter
Implements Persistent.
◆ hasIcon()
bool Node::hasIcon | ( | void | ) | const |
- Returns
- true if an instance-specific icon has been set
◆ iconHeight()
double Node::iconHeight | ( | void | ) | const |
- Returns
- the heigth in px of the icon classes derived from VertexBase can override it by calling setIcon in the constructor
- See also
- Layout
◆ iconName()
std::string Node::iconName | ( | bool | raster | ) | const |
- Returns
- the name of the icon, either the static one or the instance-specific one if it has been set
- Parameters
-
raster if true, returns raster (png) icons, else they vector (svg) icons
- See also
- Layout
◆ iconWidth()
double Node::iconWidth | ( | void | ) | const |
- Returns
- the width in px of the icon classes derived from VertexBase can override it by calling setIcon in the constructor
- See also
- Layout
◆ insert()
|
overridevirtual |
auto-determine the offset and recursively insert Node into persistency
- Returns
- the database id of the inserted Node
- Parameters
-
persistency database connection
Implements Persistent.
◆ isRestored()
bool Node::isRestored | ( | void | ) | const |
return whether the Node has just been retrieved from persistent storage
◆ offset()
|
inlineprotected |
◆ operator!=()
bool Node::operator!= | ( | const Node & | rhs | ) | const |
- Returns
- true if the two Nodes are recursively different
◆ operator=() [1/2]
copy assignment operator copies all elements but root which is set to nullptr
Reimplemented in Object.
◆ operator=() [2/2]
◆ operator==()
bool Node::operator== | ( | const Node & | rhs | ) | const |
- Returns
- true if the two Nodes are recursively identical
◆ printJson()
|
virtual |
◆ prune()
std::unique_ptr< Node > Node::prune | ( | const std::string & | tag | ) |
removes the Node with human-readable label tag, and all its descendants
- Returns
- the unique pointer to the pruned Node, can be grafted somewhere else or discarded, in this case the pointed-to tree will be destroyed
- Parameters
-
tag UTF-8 encoded human-readable label
- Exceptions
-
if there no child with the supplied label tag
◆ range()
|
overridevirtual |
- Returns
- the range of ids required to save to persistency this Node and all its descendants; minimum value is 0
Implements Persistent.
◆ readParameters()
|
inlinevirtual |
reads I, IV, S and SV from persistency
Reimplemented in Object.
◆ readVariables()
|
inlinevirtual |
reads Q, QV and QM from persistency
Reimplemented in Object.
◆ remove()
|
overridevirtual |
remove object from persistency database
- Parameters
-
persistency database connection
Implements Persistent.
◆ renameChild()
void Node::renameChild | ( | const std::string & | oldName, |
const std::string & | newName | ||
) |
renames child (direct descendant)
- Parameters
-
[in] oldName old child name [in] newName new child name
◆ restore()
|
overridevirtual |
recursively restore Node variable values from persistency
- Parameters
-
persistency database connection
Implements Persistent.
◆ root()
|
inline |
- Returns
- a pointer to the root Persistent object
◆ rootId()
|
overridevirtual |
- Returns
- the unique integer identifier of the root item
Implements Persistent.
◆ search()
Node & Node::search | ( | uint32_t | id | ) |
recursively looks for a child with the supplied id
- Exceptions
-
if no match is found
◆ setIcon()
void Node::setIcon | ( | std::string | icon, |
double | width, | ||
double | height | ||
) |
change icon for the instance
- Parameters
-
[in] icon the icon name without extension i.e. "Valve" [in] width the icon width for SVG output [in] height the icon height for SVG output
- See also
- Layout
◆ type()
|
overridevirtual |
- Returns
- the C++ name of the class the instance belongs to
- Note
- it is the responsibility of the caller to not access this reference after the lifecycle of the Item
Implements Item.
Reimplemented in Object, PhaseActivity< U >, PhaseGeneric< T >, PhaseGeneric< U >, PhaseSimple, PhaseSimpleTotal, PhaseTotal, StreamIapwsLiquidVapor, StreamOne, StreamOneSolid, StreamSimple< NV, NL, NS >, StreamTabularLiquidLiquid, StreamTabularLiquidVapor, StreamTwo< F >, StreamTwo< FlashVl >, StreamTwoSolid< F >, Compressor, Degasser, Divider, Ejector, Exchanger, FlashDegasser, FlashDrum, FlashSplitter, HeatTransfer, HtuNtu, Mixer, MultiExchanger, Multiplier, MultiReaction< N >, MultiReaction< 2 >, MultiReactionElectrochemical, MultiReactionTransfer, Pipe, Pump, RatingHeat, RatingHeatShellAndTubeFallingFilmReboiler, RatingHeatShellAndTubeHeater, RatingHeatShellAndTubeRecovery, RatingHeatShellAndTubeThermosiphon, Reaction, ReactionEquilibrium, ReactionOxidationHydrocarbon, ReactionWaterGasShift, ReactionWaterGasShiftEquilibrium, ReactionOxidationEquilibriumCH4, ReactionOxidationCO, ReactionWaterGasC, ReactionReformingCH4, ReactionReformingEquilibriumCH4, ReactionReformingC2H6, ReactionReformingEquilibriumC2H6, ReactionReformingC3H8, ReactionReformingEquilibriumC3H8, ReactionOxidationMeOH, ReactionReformingMeOH, ReactionReformingEquilibriumMeOH, ReactionSynthesisNH3, ReactionSynthesisEquilibriumNH3, ReactionOxidationN2, ReactionOxidationEquilibriumN2, ReactionOxidationC2H6, ReactionOxidationNH3, ReactionOxidationPhenol, ReactionYield, Selector, Separator, ShellAndTube, and Splitter.
◆ update()
|
overridevirtual |
recursively update Node into persistency
- Returns
- the database id of the updated Node
- Parameters
-
persistency database connection
Implements Persistent.
Friends And Related Function Documentation
◆ Iterator
|
friend |
◆ Object
|
friend |
◆ operator<<
|
friend |
Member Data Documentation
◆ children_
|
protected |
the collection of direct descendants
The documentation for this class was generated from the following file: