A Node with Quantity, String and Integer variables. More...
#include <Object.h>
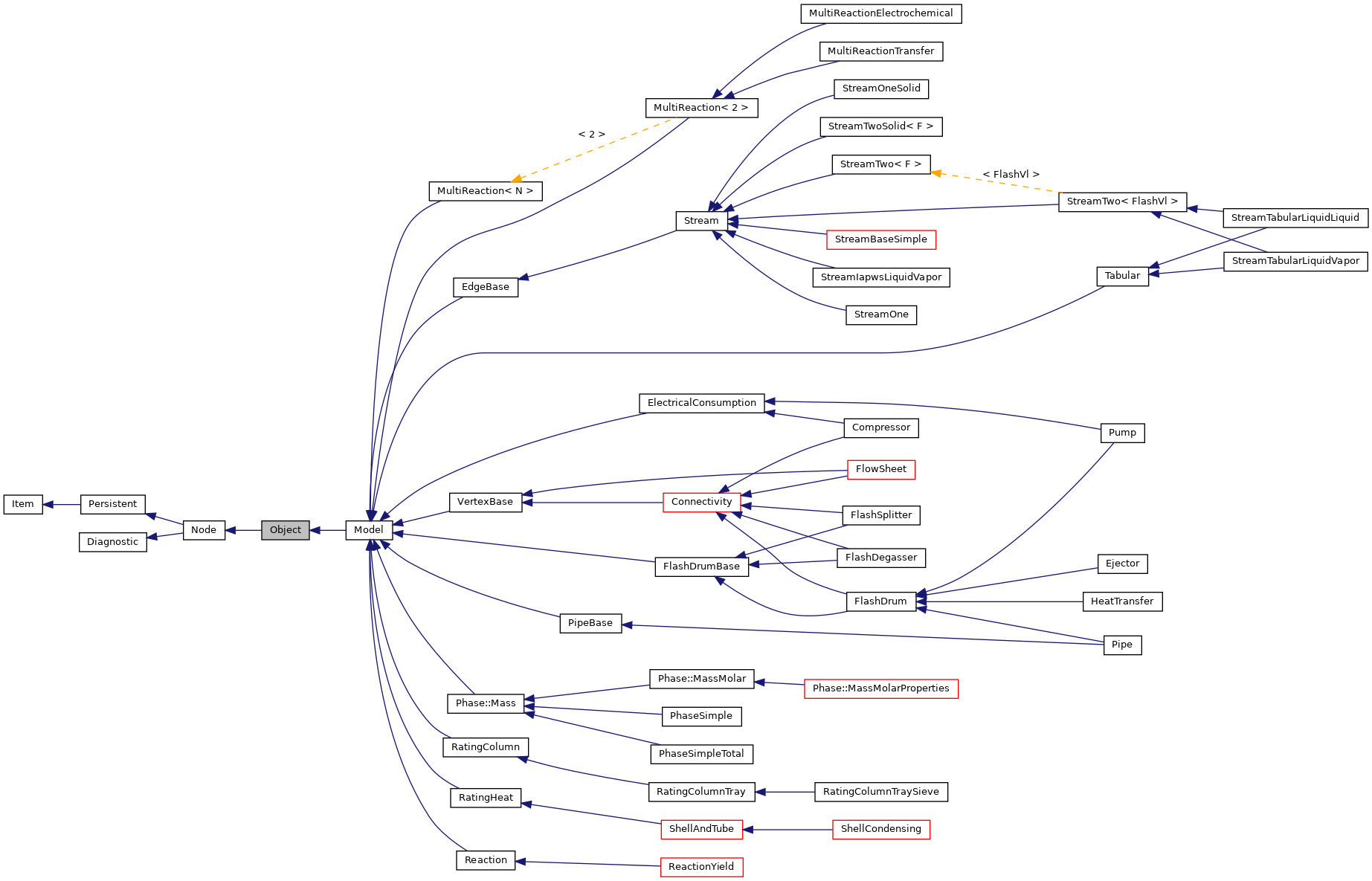
Classes | |
class | ConstIteratorQuantities |
class | ConstRangeQuantities |
class | IteratorQuantities |
class | RangeQuantities |
Public Member Functions | |
Object (Libpf::Persistency::Defaults defaults, uint32_t id=0, Persistency *persistency=nullptr, Persistent *parent=nullptr, Persistent *root=nullptr) | |
Libpf::Persistency::Defaults | defaults (void) const |
std::ostream & | printJson (std::ostream &os, int level=0) const override |
print Node in JSON format More... | |
void | readVariables (Persistency *persistency) override |
reads Q, QV and QM from persistency More... | |
void | readParameters (Persistency *persistency) override |
reads I, IV, S and SV from persistency More... | |
Node & | operator= (const Node &) override |
copy assignment operator More... | |
Node & | operator= (Node &&other) override |
move assignment More... | |
~Object (void) | |
const std::string & | type (void) const override |
Object & | at (const std::string &fullRelativeTag) |
const Object & | at (const std::string &fullRelativeTag) const |
Object & | at (const std::string &fullRelativeTag, int index) |
const Value & | Q (const std::string &tag) const |
const Value & | Q (const std::string &tag, int index) const |
const Value & | Q (const std::string &tag, int row, int column) const |
Value & | Q (const std::string &tag) |
Value & | Q (const std::string &tag, int index) |
Value & | Q (const std::string &tag, int row, int column) |
const Value & | Q (const std::string &tag, std::string componentName) const |
Value & | Q (const std::string &tag, std::string componentName) |
const Value & | Q (const std::string &tag, int index, std::string componentName) const |
Value & | Q (const std::string &tag, int index, std::string componentName) |
const int & | I (const std::string &tag) const |
const int & | I (const std::string &tag, int index) const |
int & | I (const std::string &tag) |
int & | I (const std::string &tag, int index) |
const std::string & | S (const std::string &tag) const |
const std::string & | S (const std::string &tag, int index) const |
std::string & | S (const std::string &tag) |
std::string & | S (const std::string &tag, int index) |
bool | operator!= (const Object &rhs) const |
bool | operator== (const Object &rhs) const |
bool | compareParameters (const Object &rhs) const |
bool | existsI (const std::string &tag) const |
bool | existsS (const std::string &tag) const |
bool | existsQ (const std::string &tag) const |
void | push (void) const |
store the current status of the object and all its sub-objects in the last-in-first-out queue of real variables values More... | |
void | pop (void) |
restore the status of the object and all its sub-objects from the last-in-first-out queue of real variables values More... | |
void | clear (void) const |
clear the last-in-first-out queue of real variables values used for storing and retrieving the status More... | |
const RangeQuantities | quantities (void) |
const ConstRangeQuantities | quantities (void) const |
![]() | |
Node (Libpf::Persistency::Defaults defaults, uint32_t id=0, Persistency *persistency=nullptr, Persistent *parent=nullptr, Persistent *root=nullptr) | |
Node (const Node &other) | |
copy constructor More... | |
Node (Node &&other) | |
move constructor More... | |
virtual std::unique_ptr< Node > | clone (void) const |
virtual Node & | operator= (const Node &other) |
copy assignment operator copies all elements but root which is set to nullptr More... | |
virtual Node & | operator= (Node &&other) |
move assignment More... | |
~Node () | |
int | insert (Persistency *persistency) const override |
int | update (Persistency *persistency) const override |
void | restore (Persistency *persistency) override |
void | remove (Persistency *persistency) const override |
uint32_t | rootId (void) const override |
uint32_t | range (void) const override |
uint32_t | getId (void) override |
returns the next available id and increments the internal counter More... | |
const std::string & | type (void) const override |
virtual std::ostream & | printJson (std::ostream &os, int level=0) const |
print Node in JSON format More... | |
Node & | addChild (std::string type, uint32_t id, Persistency *persistency) |
Node & | addChild (std::string type, Libpf::Persistency::Defaults defaults) |
Node & | addChild (const Node &child) |
Node & | addChild (std::unique_ptr< Node > child) |
std::unique_ptr< Node > | prune (const std::string &tag) |
const Node & | child (const std::string &tag) const |
Node & | at (const std::string &fullRelativeTag) |
const Node & | at (const std::string &fullRelativeTag) const |
uint32_t | descendants (void) const |
Persistent * | root (void) const |
void | renameChild (const std::string &oldName, const std::string &newName) |
bool | existsChild (std::string tag) const |
bool | exists (uint32_t id) |
Node & | search (uint32_t id) |
bool | operator!= (const Node &rhs) const |
bool | operator== (const Node &rhs) const |
virtual void | readVariables (Persistency *) |
reads Q, QV and QM from persistency More... | |
virtual void | readParameters (Persistency *) |
reads I, IV, S and SV from persistency More... | |
bool | isRestored (void) const |
return whether the Node has just been retrieved from persistent storage More... | |
void | setIcon (std::string icon, double width, double height) |
bool | hasIcon (void) const |
std::string | iconName (bool raster) const |
double | iconWidth (void) const |
double | iconHeight (void) const |
const Range | children (void) |
const ConstRange | children (void) const |
![]() | |
Persistent (const std::string &tag, const std::string &description, Persistent *parent, uint32_t id) | |
main constructor More... | |
Persistent (const Persistent &) | |
copy constructor More... | |
Persistent & | operator= (const Persistent &) |
copy assignment More... | |
Persistent (Persistent &&other) | |
move constructor More... | |
Persistent & | operator= (Persistent &&other) |
move assignment More... | |
~Persistent (void) | |
uint32_t | id (void) const |
std::string | uuid (void) const |
uint32_t | parentId (void) const |
virtual uint32_t | rootId (void) const =0 |
virtual uint32_t | range (void) const =0 |
virtual uint32_t | getId (void)=0 |
returns the next available id and increments the internal counter More... | |
double | created_at (void) const |
double | updated_at (void) const |
void | updated_at (double u) const |
virtual int | insert (Persistency *persistency) const =0 |
virtual int | update (Persistency *persistency) const =0 |
virtual void | restore (Persistency *persistency)=0 |
virtual void | remove (Persistency *persistency) const =0 |
![]() | |
Item (const Item &other) | |
copy constructor copies all elements but parent which is set to nullptr More... | |
Item & | operator= (const Item &other) |
copy assignment operator copies all elements but parent which is set to nullptr More... | |
Item (const std::string &tag, const std::string &description, Persistent *parent) | |
Item (Item &&other) | |
move constructor More... | |
Item & | operator= (Item &&other) |
move assignment operator More... | |
virtual | ~Item () |
const std::string & | tag () const |
const std::string & | description () const |
std::string | fullTag () const |
const Persistent * | parent () const |
virtual const std::string & | type () const =0 |
void | setTag (const std::string &tag) |
void | setDescription (const std::string &description) |
void | setWideTag (const std::wstring &tag) |
wide-character variant, More... | |
void | setWideDescription (const std::wstring &description) |
wide-character variant, More... | |
bool | operator!= (const Item &rhs) const |
bool | operator== (const Item &rhs) const |
![]() | |
Diagnostic (void) | |
void | setVerbosity (int verbosity) |
sets the verbosity level for this instance More... | |
int | verbosity (void) const |
Protected Member Functions | |
int | retrieveInteger (const Libpf::Persistency::Defaults &defaults, uint32_t id, Persistency *persistency, std::string tag, int min, int max, int def=-1) |
std::string | retrieveString (const Libpf::Persistency::Defaults &defaults, uint32_t id, Persistency *persistency, std::string tag, const std::string def) |
template<class T > | |
void | addVariable (T &variable) |
template<class T > | |
void | addVectorVariable (std::vector< T > &variable, const std::string &tag, const std::string &description, uint32_t size, typename T::ValueType value) |
Object (const Object &other) | |
copy constructor More... | |
Object (Object &&other) | |
move constructor More... | |
Object & | operator= (const Object &) |
copy assignment operator More... | |
Object & | operator= (Object &&other) |
move assignment operator More... | |
![]() | |
int | offset (void) const |
![]() | |
virtual | ~Diagnostic ()=default |
Protected Attributes | |
std::map< std::string, Integer * > | integers_ |
collection of integer variables More... | |
std::map< std::string, IntegerVector * > | integerVectors_ |
collection of integer variable vectors More... | |
std::map< std::string, Quantity * > | quantities_ |
collection of real variables More... | |
std::map< std::string, QuantityVector * > | quantityVectors_ |
collection of real variable vectors More... | |
std::map< std::string, QuantityMatrix * > | quantityMatrices_ |
collection of real variable matrices More... | |
std::map< std::string, String * > | strings_ |
collection of string variables More... | |
std::map< std::string, StringVector * > | stringVectors_ |
collection of string variable vectors More... | |
![]() | |
std::map< std::string, std::unique_ptr< Node > > | children_ |
the collection of direct descendants More... | |
![]() | |
Persistent * | parent_ |
pointer to the parent Persistent; set to this by the constructor if Item is self-standing or a root Persistent of a tree More... | |
![]() | |
int | verbosityInstance |
Detailed Description
Constructor & Destructor Documentation
◆ Object() [1/3]
|
protected |
copy constructor
◆ Object() [2/3]
|
protected |
move constructor
◆ Object() [3/3]
Object::Object | ( | Libpf::Persistency::Defaults | defaults, |
uint32_t | id = 0 , |
||
Persistency * | persistency = nullptr , |
||
Persistent * | parent = nullptr , |
||
Persistent * | root = nullptr |
||
) |
universal constructor
- Parameters
-
defaults default informations to build and configure the Node and its descendants id integer identifier, for a Node belonging to a tree must be unique within the tree; defaults to 0 persistency database connection parent pointer to the parent Node; set to nullptr if Node is self-standing or the root Node in a tree; defaults to nullptr root pointer to the root Node; set to nullptr if Node is self-standing or the root Node in a tree; defaults to nullptr
◆ ~Object()
Object::~Object | ( | void | ) |
Member Function Documentation
◆ addVariable()
|
protected |
inserts a reference to an automatic variable in the collections of variables
- Parameters
-
variable the automatic variable
◆ addVectorVariable()
|
protected |
populates a vector variable and inserts the references in the collections of variables
- Exceptions
-
ErrorRunTime if there is already a variable with the same label tag
- Parameters
-
variable the vector variable tag the tag of the vector variable description the description of the vector variable size the size of the vector variable value the initialization value for the elements of the vector variable
◆ at() [1/3]
Object & Object::at | ( | const std::string & | fullRelativeTag | ) |
- Returns
- a reference to the recursive descendant with the supplied full relative label
- Parameters
-
fullRelativeTag the UTF-8 encoded full relative human-readable label, unique within the scope of this Node and all its descendants
- Exceptions
-
ErrorRunTime if there no descendant with the supplied full relative label tag
- Note
- it is the responsibility of the caller to not access this reference after the lifecycle of the Object is over
◆ at() [2/3]
const Object & Object::at | ( | const std::string & | fullRelativeTag | ) | const |
- Returns
- a const reference to the recursive descendant with the supplied full relative label
- Parameters
-
fullRelativeTag the UTF-8 encoded full relative human-readable label, unique within the scope of this Node and all its descendants
- Note
- it is the responsibility of the caller to not access this reference after the lifecycle of the Node is over
- Exceptions
-
ErrorRunTime if there no descendant with the supplied full relative label tag
◆ at() [3/3]
Object & Object::at | ( | const std::string & | fullRelativeTag, |
int | index | ||
) |
- Returns
- a reference to the recursive descendant corresponding to the supplied full relative tag and index in the collection of embedded objects
- Parameters
-
fullRelativeTag the UTF-8 encoded full relative human-readable label, unique within the scope of this Node and all its descendants index index of element to retrieve, 0-base
- Note
- it is the responsibility of the caller to not access this reference after the lifecycle of the Node is over
- Exceptions
-
ErrorRunTime if there no descendant vector with the supplied full relative label tag ErrorRunTime if the index is outside range
◆ clear()
void Object::clear | ( | void | ) | const |
clear the last-in-first-out queue of real variables values used for storing and retrieving the status
◆ compareParameters()
bool Object::compareParameters | ( | const Object & | rhs | ) | const |
compares Integers, Strings and vectors / matrices thereof
- Returns
- true if the two Object is recursively identical to rhs w.r.t. parameters
◆ defaults()
Libpf::Persistency::Defaults Object::defaults | ( | void | ) | const |
- Returns
- the information originally supplied to the constructor and persisted in Integers and Strings
◆ existsI()
bool Object::existsI | ( | const std::string & | tag | ) | const |
- Returns
- true if an element with the supplied tag exists in the collection of integer variables
- Parameters
-
tag UTF-8 encoded human-readable identifier
◆ existsQ()
bool Object::existsQ | ( | const std::string & | tag | ) | const |
- Returns
- true if an element with the supplied tag exists in the collection of real variables
- Parameters
-
tag UTF-8 encoded human-readable identifier
◆ existsS()
bool Object::existsS | ( | const std::string & | tag | ) | const |
- Returns
- true if an element with the supplied tag exists in the collection of strings
- Parameters
-
tag UTF-8 encoded human-readable identifier
◆ I() [1/4]
int & Object::I | ( | const std::string & | tag | ) |
- Returns
- a non-const reference to the integral value of the element corresponding to the supplied tag in the collection of integer variables, parameters and integer pointers to embedded object's CATALOGID
- Exceptions
-
ErrorBrowsing in case non-existing tag
- Parameters
-
tag tag of element to retrieve
- See also
- integers_
◆ I() [2/4]
const int & Object::I | ( | const std::string & | tag | ) | const |
- Returns
- a const reference to the integral value of the element corresponding to the supplied tag in the collection of integer variables, parameters and integer pointers to embedded object's CATALOGID
- Exceptions
-
ErrorBrowsing in case non-existing tag
- Parameters
-
tag tag of element to retrieve
- See also
- integers_
◆ I() [3/4]
int & Object::I | ( | const std::string & | tag, |
int | index | ||
) |
- Returns
- a non-const reference to the integral value of the i-th element from the vector with supplied tag in the collection of integer variables, parameters and integer pointers to embedded object's CATALOGID
- Exceptions
-
ErrorBrowsing in case non-existing tag
- Parameters
-
tag tag of element to retrieve index index of element to retrieve, 0-base
- See also
- integers_
◆ I() [4/4]
const int & Object::I | ( | const std::string & | tag, |
int | index | ||
) | const |
- Returns
- a const reference to the integral value of the i-th element from the vector with supplied tag in the collection of integer variables, parameters and integer pointers to embedded object's CATALOGID
- Exceptions
-
ErrorBrowsing in case non-existing tag
- Parameters
-
tag tag of element to retrieve index index of element to retrieve, 0-base
- See also
- integers_
◆ operator!=()
bool Object::operator!= | ( | const Object & | rhs | ) | const |
- Returns
- true if the two Objects are recursively different
◆ operator=() [1/4]
copy assignment operator
Reimplemented from Node.
◆ operator=() [2/4]
◆ operator=() [3/4]
◆ operator=() [4/4]
◆ operator==()
bool Object::operator== | ( | const Object & | rhs | ) | const |
- Returns
- true if the two Objects are recursively identical
◆ pop()
void Object::pop | ( | void | ) |
restore the status of the object and all its sub-objects from the last-in-first-out queue of real variables values
◆ printJson()
|
overridevirtual |
◆ push()
void Object::push | ( | void | ) | const |
store the current status of the object and all its sub-objects in the last-in-first-out queue of real variables values
◆ Q() [1/10]
Value & Object::Q | ( | const std::string & | tag | ) |
- Returns
- a non-const reference to the real value of the element corresponding to the supplied tag in the collection of real variables
- Exceptions
-
ErrorBrowsing in case non-existing tag
- Parameters
-
tag label of element to retrieve
- See also
- quantities_
◆ Q() [2/10]
const Value & Object::Q | ( | const std::string & | tag | ) | const |
- Returns
- a const reference to the real value of the element corresponding to the supplied tag in the collection of real variables
- Exceptions
-
ErrorBrowsing in case non-existing tag
- Parameters
-
tag label of element to retrieve
- See also
- quantities_
◆ Q() [3/10]
Value & Object::Q | ( | const std::string & | tag, |
int | index | ||
) |
- Returns
- a non-const reference to the real value of the element corresponding to the supplied tag and index in the collection of real variable vectors
- Exceptions
-
ErrorBrowsing in case non-existing tag
- Parameters
-
tag label of vector element index index of element to retrieve, 0-base
- See also
- quantities_
◆ Q() [4/10]
const Value & Object::Q | ( | const std::string & | tag, |
int | index | ||
) | const |
- Returns
- a const reference to the real value of the element corresponding to the supplied tag and index in the collection of real variable vectors
- Exceptions
-
ErrorBrowsing in case non-existing tag
- Parameters
-
tag label of vector element index index of element to retrieve, 0-base
- See also
- quantities_
◆ Q() [5/10]
Value & Object::Q | ( | const std::string & | tag, |
int | index, | ||
std::string | componentName | ||
) |
- Returns
- a non-const reference to the real value of the element corresponding to the supplied tag, index and component in the collection of real variable matrices
- Exceptions
-
ErrorBrowsing in case non-existing tag
- Parameters
-
tag label of vector element index index of element to retrieve, 0-base componentName component name of vector element to retrieve
- See also
- quantities_
◆ Q() [6/10]
const Value & Object::Q | ( | const std::string & | tag, |
int | index, | ||
std::string | componentName | ||
) | const |
- Returns
- a const reference to the real value of the element corresponding to the supplied tag, index and component in the collection of real variable matrices
- Exceptions
-
ErrorBrowsing in case non-existing tag
- Parameters
-
tag label of vector element index index of element to retrieve, 0-base componentName component name of vector element to retrieve
- See also
- quantities_
◆ Q() [7/10]
Value & Object::Q | ( | const std::string & | tag, |
int | row, | ||
int | column | ||
) |
- Returns
- a non-const reference to the real value of the element corresponding to the supplied tag, row and column in the collection of real variable matrices
- Exceptions
-
ErrorBrowsing in case non-existing tag
- Parameters
-
tag label of vector element row row index of element to retrieve, 0-base column column index of element to retrieve, 0-base
- See also
- quantities_
◆ Q() [8/10]
const Value & Object::Q | ( | const std::string & | tag, |
int | row, | ||
int | column | ||
) | const |
- Returns
- a const reference to the real value of the element corresponding to the supplied tag, row and column in the collection of real variable matrices
- Exceptions
-
ErrorBrowsing in case non-existing tag
- Parameters
-
tag label of vector element row row index of element to retrieve, 0-base column column index of element to retrieve, 0-base
- See also
- quantities_
◆ Q() [9/10]
Value & Object::Q | ( | const std::string & | tag, |
std::string | componentName | ||
) |
- Returns
- a non-const reference to the real value of the element with the supplied component name from a dictionary with supplied tag in the collection of real variables
- Exceptions
-
ErrorBrowsing in case non-existing tag
- Parameters
-
tag label of vector element componentName component name of vector element to retrieve
- See also
- quantities_
◆ Q() [10/10]
const Value & Object::Q | ( | const std::string & | tag, |
std::string | componentName | ||
) | const |
- Returns
- a const reference to the real value of the element with the supplied component name from a dictionary with supplied tag in the collection of real variables
- Exceptions
-
ErrorBrowsing in case non-existing tag
- Parameters
-
tag label of vector element componentName component name of vector element to retrieve
- See also
- quantities_
◆ quantities() [1/2]
const RangeQuantities Object::quantities | ( | void | ) |
- Returns
- a RangeQuantities object that with a range-based for-loop can iterate over all real variables
◆ quantities() [2/2]
const ConstRangeQuantities Object::quantities | ( | void | ) | const |
- Returns
- a ConstRangeQuantities object that with a const range-based for-loop can iterate over all real variables
◆ readParameters()
|
overridevirtual |
reads I, IV, S and SV from persistency
Reimplemented from Node.
◆ readVariables()
|
overridevirtual |
reads Q, QV and QM from persistency
Reimplemented from Node.
◆ retrieveInteger()
|
protected |
retrieve a strictly positive configuration integer parameter if persistency is nullptr, get it from defaults; else try to retrieve it from persistency based on id if the supplied / retrieved value is negative, set it to default value; in any case make sure the final value is strictly within bounds
- Parameters
-
defaults defaults passed to constructor id integer identifier, for a Node belonging to a tree must be unique within the tree; defaults to 0 persistency database connection tag parameter name min parameter minimum value (strict: can not be equal) max parameter maximum value (strict: can not be equal) def default value if no user input is present in options
◆ retrieveString()
|
protected |
retrieve a non-empty configuration string parameter if persistency is nullptr, get it from defaults else try to retrieve it from persistency based on id if the retrieved value is empty, set it to default value
- Parameters
-
defaults defaults passed to constructor id integer identifier, for a Node belonging to a tree must be unique within the tree; defaults to 0 persistency database connection tag parameter name def default value if no user input is present in options
◆ S() [1/4]
std::string & Object::S | ( | const std::string & | tag | ) |
- Returns
- a non-const reference to the string value of the element corresponding to the supplied tag in the collection of strings
- Exceptions
-
ErrorBrowsing in case non-existing tag
- Parameters
-
tag tag of element to retrieve
- See also
- strings_
◆ S() [2/4]
const std::string & Object::S | ( | const std::string & | tag | ) | const |
- Returns
- a const reference to the string value of the element corresponding to the supplied tag in the collection of strings
- Exceptions
-
ErrorBrowsing in case non-existing tag
- Parameters
-
tag tag of element to retrieve
- See also
- strings_
◆ S() [3/4]
std::string & Object::S | ( | const std::string & | tag, |
int | index | ||
) |
- Returns
- a non-const reference to the string value of the i-th element from the vector with supplied tag in the collection of strings
- Exceptions
-
ErrorBrowsing in case non-existing tag
- Parameters
-
tag tag of element to retrieve index index of element to retrieve, 0-base
- See also
- strings_
◆ S() [4/4]
const std::string & Object::S | ( | const std::string & | tag, |
int | index | ||
) | const |
- Returns
- a reference to the string value of the i-th element from the vector with supplied tag in the collection of strings
- Exceptions
-
ErrorBrowsing in case non-existing tag
- Parameters
-
tag tag of element to retrieve index index of element to retrieve, 0-base
- See also
- strings_
◆ type()
|
overridevirtual |
- Returns
- the C++ name of the class the instance belongs to
- Note
- it is the responsibility of the caller to not access this reference after the lifecycle of the Item
Reimplemented from Node.
Reimplemented in PhaseActivity< U >, PhaseGeneric< T >, PhaseGeneric< U >, PhaseSimple, PhaseSimpleTotal, PhaseTotal, StreamIapwsLiquidVapor, StreamOne, StreamOneSolid, StreamSimple< NV, NL, NS >, StreamTabularLiquidLiquid, StreamTabularLiquidVapor, StreamTwo< F >, StreamTwo< FlashVl >, StreamTwoSolid< F >, Compressor, Degasser, Divider, Ejector, Exchanger, FlashDegasser, FlashDrum, FlashSplitter, HeatTransfer, HtuNtu, Mixer, MultiExchanger, Multiplier, MultiReaction< N >, MultiReaction< 2 >, MultiReactionElectrochemical, MultiReactionTransfer, Pipe, Pump, RatingHeat, RatingHeatShellAndTubeFallingFilmReboiler, RatingHeatShellAndTubeHeater, RatingHeatShellAndTubeRecovery, RatingHeatShellAndTubeThermosiphon, Reaction, ReactionEquilibrium, ReactionOxidationHydrocarbon, ReactionWaterGasShift, ReactionWaterGasShiftEquilibrium, ReactionOxidationEquilibriumCH4, ReactionOxidationCO, ReactionWaterGasC, ReactionReformingCH4, ReactionReformingEquilibriumCH4, ReactionReformingC2H6, ReactionReformingEquilibriumC2H6, ReactionReformingC3H8, ReactionReformingEquilibriumC3H8, ReactionOxidationMeOH, ReactionReformingMeOH, ReactionReformingEquilibriumMeOH, ReactionSynthesisNH3, ReactionSynthesisEquilibriumNH3, ReactionOxidationN2, ReactionOxidationEquilibriumN2, ReactionOxidationC2H6, ReactionOxidationNH3, ReactionOxidationPhenol, ReactionYield, Selector, Separator, ShellAndTube, and Splitter.
Member Data Documentation
◆ integers_
|
protected |
collection of integer variables
◆ integerVectors_
|
protected |
collection of integer variable vectors
◆ quantities_
|
protected |
collection of real variables
◆ quantityMatrices_
|
protected |
collection of real variable matrices
◆ quantityVectors_
|
protected |
collection of real variable vectors
◆ strings_
|
protected |
collection of string variables
◆ stringVectors_
|
protected |
collection of string variable vectors
The documentation for this class was generated from the following file: