Pesistent storage management class. More...
#include <Persistency.h>
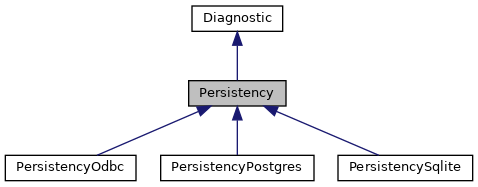
Public Member Functions | |
Persistency (void) | |
virtual | ~Persistency (void) |
terminates connection to database More... | |
virtual void | push_transaction (void)=0 |
increment transaction depth More... | |
virtual void | pop_transaction (void)=0 |
decrement transaction depth; if depth reaches zero, commit More... | |
int | transaction_depth (void) |
virtual void | purge (const std::string &tablename, int i=-1)=0 |
void | purgeAll (void) |
purges all tables, N as last More... | |
virtual int | countRows (const std::string &tablename, int i=-1)=0 |
virtual int | getId (void)=0 |
virtual void | remove (int i, const std::string &tablename)=0 |
virtual int | countCID (int i, const std::string &)=0 |
virtual int | insertObject (const Persistent &object, int offset)=0 |
virtual void | retrieveObject (int id, std::string &uuid, std::string &tag, std::string &description, std::string &type, int &parentId, int &rootId, double &created_at, double &modified_at)=0 |
retrieves the node based on the id pseudocode for SQL query is SELECT UUID, TAG, DESCRIPTION, TYPE, PARENT, ROOT FROM N WHERE ID = id More... | |
virtual void | retrieveObject (const std::string &uuid, int &id, std::string &tag, std::string &description, std::string &type, int &parentId, int &rootId, double &created_at, double &modified_at)=0 |
retrieves the node based on the uuid pseudocode for SQL query is SELECT ID, TAG, DESCRIPTION, TYPE, PARENT, ROOT FROM N WHERE UUID = uuid More... | |
virtual void | retrieveChildren (int id, std::vector< std::pair< int, std::string > > &children)=0 |
retrieves the node's children based on the id pseudocode for SQL query is SELECT ID, TYPE FROM N WHERE PARENT = id ORDER BY ID More... | |
virtual void | removeObject (int id)=0 |
remove object from persistency database pseudocode for SQL query is: DELETE FROM {IVV,SVV,QVV,QMV} JOIN {IV,SV,QV,QM} ON IVV.VID = IV.ID WHERE IV.NID IN [id, id+RANGE] DELETE FROM {I,IV,S,SV,Q,QV,QM} WHERE NID IN [id, id+RANGE] DELETE FROM N WHERE ID IN [id, id+RANGE] More... | |
virtual void | update (int id)=0 |
virtual bool | try_lock (int id, std::string &user, int duration)=0 |
virtual bool | check_lock (int id, std::string &user)=0 |
virtual bool | release_lock (int id, std::string &user)=0 |
virtual void | insert (const std::map< std::string, Integer * > &elements, int offset)=0 |
virtual void | read (int id, std::map< std::string, Integer * > &elements)=0 |
virtual void | update (int id, const std::map< std::string, Integer * > &elements)=0 |
int | readInteger (int id, const std::string &tag) |
virtual void | insert (const std::map< std::string, Quantity * > &elements, int offset)=0 |
virtual void | read (int id, std::map< std::string, Quantity * > &elements)=0 |
virtual void | update (int id, const std::map< std::string, Quantity * > &elements)=0 |
virtual void | insert (const std::map< std::string, String * > &elements, int offset)=0 |
virtual void | read (int id, std::map< std::string, String * > &elements)=0 |
virtual void | update (int id, const std::map< std::string, String * > &elements)=0 |
std::string | readString (int id, const std::string &tag) |
virtual void | insert (const std::map< std::string, IntegerVector * > &elements, int offset)=0 |
virtual void | read (int id, std::map< std::string, IntegerVector * > &elements)=0 |
virtual void | update (int id, const std::map< std::string, IntegerVector * > &elements)=0 |
virtual void | insert (const std::map< std::string, QuantityVector * > &elements, int offset)=0 |
virtual void | read (int id, std::map< std::string, QuantityVector * > &elements)=0 |
virtual void | update (int id, const std::map< std::string, QuantityVector * > &elements)=0 |
virtual void | insert (const std::map< std::string, QuantityMatrix * > &elements, int offset)=0 |
virtual void | read (int id, std::map< std::string, QuantityMatrix * > &elements)=0 |
virtual void | update (int id, const std::map< std::string, QuantityMatrix * > &elements)=0 |
virtual void | insert (const std::map< std::string, StringVector * > &elements, int offset)=0 |
virtual void | read (int id, std::map< std::string, StringVector * > &elements)=0 |
virtual void | update (int id, const std::map< std::string, StringVector * > &elements)=0 |
![]() | |
Diagnostic (void) | |
void | setVerbosity (int verbosity) |
sets the verbosity level for this instance More... | |
int | verbosity (void) const |
Protected Member Functions | |
void | init (void) |
void | destroy (void) |
void | lock (void) |
void | unlock (void) |
![]() | |
virtual | ~Diagnostic ()=default |
Protected Attributes | |
int | transaction_depth_ |
![]() | |
int | verbosityInstance |
Detailed Description
Pesistent storage management class.
This class manage the memory storage of PersistenInterface objects
#include <libpf/persistency/Persistency.h>
- Remarks
- Warning
- Thread safe:
- Extendable:
- Platform dependencies:
Constructor & Destructor Documentation
◆ Persistency()
Persistency::Persistency | ( | void | ) |
When the database object is instantiated:
- it initializes once and for all the connection to the database (RAII = Resource Acquisition Is Initialization)
- stores a handle or pointer to the database connection in a non-static member Connections to many different databases can be held simutaneously active at one time.
- Exceptions
-
Error if the connection is impossible to establish
◆ ~Persistency()
|
virtual |
terminates connection to database
Member Function Documentation
◆ check_lock()
|
pure virtual |
check lock on item id
- Returns
- true if we still hold the lock, false if there is no lock or if somebody else is holding the lock
- Parameters
-
id id in N of item to lock user name of the locking user (input / output: on input, the user attempting the lock, on output unchanged if lock successful, or the user currently holding the lock if unsuccessful)
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ countCID()
|
pure virtual |
counts the records with NID == i in table tablename the argument i can only be >=-1 if it is == -1 the function returns the number of records in table tablename The SQL query is: "SELECT COUNT(*) FROM " + tablename if it is >= 0 the function returns the number of records in table tablename with NID equal to the value of the i parameter The SQL query is: "SELECT COUNT(*) FROM " + tablename + " WHERE NID = "+ i Throws an exception if table tablename does not exist.
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ countRows()
|
pure virtual |
count rows in table tablename; if i is greater or equal to zero, filter for ID == i the argument i can only be >=-1 if it is == -1 the function returns the number of records in table tablename The SQL query is: SELECT COUNT(*) FROM " + tablename if it is >= 0 the function returns the number of records in table tablename with ID equal to the value of the i parameter; since ID is a primary key for all tables, in this case only two return values are possible: 0 or 1 The SQL query is: "SELECT COUNT(*) FROM " + tablename + " WHERE ID = " + i Throws an exception if table tablename does not exist.
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ destroy()
|
protected |
◆ getId()
|
pure virtual |
- Returns
- the next available id, or 0 if no row is yet present in table N The SQL query is: "SELECT MAX(ID+range-1) FROM N"
- Exceptions
-
Error in case of error
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ init()
|
protected |
◆ insert() [1/7]
|
pure virtual |
bulk insert for Integers; uses an efficient mechanism similar to ODBC's SQLPrepare / SQLBindParameter / SQLExecute
- Note
- SQL pseudo-code: insert into I(NID, TAG, DESCRIPTION, VALUE) values(?, ?, ?, ?)
- Parameters
-
elements collection of Integer variables offset offset is added to each parent()->id() to get the NID
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ insert() [2/7]
|
pure virtual |
bulk insert for IntegerVectors; uses an efficient mechanism similar to ODBC's SQLPrepare / SQLBindParameter / SQLExecute
- Parameters
-
elements collection of IntegerVector variables offset offset is added to each parent()->id() to get the NID
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ insert() [3/7]
|
pure virtual |
bulk insert for Quantities; uses an efficient mechanism similar to ODBC's SQLPrepare / SQLBindParameter / SQLExecute
- Note
- SQL pseudo-code: insert into Q(NID, TAG, DESCRIPTION, VALUE) values(?, ?, ?, ?)
- Parameters
-
elements collection of Quantity variables offset offset is added to each parent()->id() to get the NID
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ insert() [4/7]
|
pure virtual |
bulk insert for QuantityMatrix; uses an efficient mechanism similar to ODBC's SQLPrepare / SQLBindParameter / SQLExecute
- Parameters
-
elements collection of QuantityMatrix variables offset offset is added to each parent()->id() to get the NID
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ insert() [5/7]
|
pure virtual |
bulk insert for QuantityVectors; uses an efficient mechanism similar to ODBC's SQLPrepare / SQLBindParameter / SQLExecute
- Parameters
-
elements collection of QuantityVector variables offset offset is added to each parent()->id() to get the NID
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ insert() [6/7]
|
pure virtual |
bulk insert for Strings; uses an efficient mechanism similar to ODBC's SQLPrepare / SQLBindParameter / SQLExecute
- Note
- SQL pseudo-code: insert into S(NID, TAG, DESCRIPTION, VALUE) values(?, ?, ?, ?)
- Parameters
-
elements collection of String variables offset offset is added to each parent()->id() to get the NID
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ insert() [7/7]
|
pure virtual |
bulk insert for StringVectors; uses an efficient mechanism similar to ODBC's SQLPrepare / SQLBindParameter / SQLExecute
- Parameters
-
elements collection of StringVector variables offset offset is added to each parent()->id() to get the NID
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ insertObject()
|
pure virtual |
insert object into persistency database SQL Query string: INSERT INTO N (ID, TAG, DESCRIPTION, TYPE, GID, COMPLETETAG, PARENT) VALUES (...)
- Returns
- the database ID of the new object
- Parameters
-
object the item to insert offset offset is added to o.id() and o.parentId() when inserting
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ lock()
|
protected |
◆ pop_transaction()
|
pure virtual |
decrement transaction depth; if depth reaches zero, commit
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ purge()
|
pure virtual |
Deletes all records in the table tablename if i=-1, else a filter is applied see below
- Parameters
-
tablename the name of the table i if tablename == N, delete from N where ID = 1; if tablename == TC delete from TC where finish = i; else delete from tablename where nid = 1 Do nothing if table has no record. Throws an exception if table tablename does not exist. Will be called for the [Q,I,S]TBL tables before so that the relations with the N are enforces at all times even during purging.
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ purgeAll()
void Persistency::purgeAll | ( | void | ) |
purges all tables, N as last
◆ push_transaction()
|
pure virtual |
increment transaction depth
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ read() [1/7]
|
pure virtual |
bulk read for Integers; only matching Item's values are updated, if no matching is found a warning is issued
- Note
- SQL pseudo-code: SELECT TAG, VALUE FROM I WHERE NID = id
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ read() [2/7]
|
pure virtual |
bulk read for Integers; only matching Item's values are updated, if no matching is found a warning is issued
- Parameters
-
id the database id of the current Object elements collection of IntegerVector variables
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ read() [3/7]
|
pure virtual |
bulk read for Quantities; only matching Item's values are updated, if no matching is found a warning is issued
- Note
- SQL pseudo-code: SELECT TAG, VALUE FROM Q WHERE NID = id
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ read() [4/7]
|
pure virtual |
bulk read for QuantityMatrix; only matching Item's values are updated, if no matching is found a warning is issued
- Parameters
-
id the database id of the current Object elements collection of QuantityMatrix variables
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ read() [5/7]
|
pure virtual |
bulk read for QuantityVectors; only matching Item's values are updated, if no matching is found a warning is issued
- Parameters
-
id the database id of the current Object elements collection of QuantityVector variables
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ read() [6/7]
|
pure virtual |
bulk read for Strings; only matching Item's values are updated, if no matching is found a warning is issued
- Note
- SQL pseudo-code: SELECT TAG, VALUE FROM S WHERE NID = id
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ read() [7/7]
|
pure virtual |
bulk read for StringVectors; only matching Item's values are updated, if no matching is found a warning is issued
- Parameters
-
id the database id of the current Object elements collection of StringVector variables
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ readInteger()
int Persistency::readInteger | ( | int | id, |
const std::string & | tag | ||
) |
individual read for Integers
- Note
- SQL pseudo-code: SELECT TAG, VALUE FROM I WHERE NID = id and TAG = tag
- Parameters
-
id the database id of the current Object tag tag of the integer variable to read
◆ readString()
std::string Persistency::readString | ( | int | id, |
const std::string & | tag | ||
) |
individual read for Strings
- Note
- SQL pseudo-code: SELECT TAG, VALUE FROM S WHERE NID = id and TAG = tag
- Parameters
-
id the database id of the current Object tag tag of the string variable to read
◆ release_lock()
|
pure virtual |
release lock on item id
- Returns
- true if we were still holding the lock, false if not
- Exceptions
-
ErrorRunTime if we hold a lock on a parent object or if the lock cannot be released for any reason
- Parameters
-
id id in N of item to lock user name of the locking user (input / output: on input, the user attempting the lock, on output unchanged if lock successful, or the user currently holding the lock if unsuccessful)
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ remove()
|
pure virtual |
removes the record with id == i in table tablename The SQL query is: "DELETE FROM " + tablename + " WHERE ID = " + i Throws an exception if table tablename does not exist.
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ removeObject()
|
pure virtual |
remove object from persistency database pseudocode for SQL query is: DELETE FROM {IVV,SVV,QVV,QMV} JOIN {IV,SV,QV,QM} ON IVV.VID = IV.ID WHERE IV.NID IN [id, id+RANGE] DELETE FROM {I,IV,S,SV,Q,QV,QM} WHERE NID IN [id, id+RANGE] DELETE FROM N WHERE ID IN [id, id+RANGE]
- Parameters
-
[in] id the id of the node to retrieve
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ retrieveChildren()
|
pure virtual |
retrieves the node's children based on the id pseudocode for SQL query is SELECT ID, TYPE FROM N WHERE PARENT = id ORDER BY ID
- Parameters
-
[in] id the id of the node to retrieve [out] children a vector of child ids and types
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ retrieveObject() [1/2]
|
pure virtual |
retrieves the node based on the uuid pseudocode for SQL query is SELECT ID, TAG, DESCRIPTION, TYPE, PARENT, ROOT FROM N WHERE UUID = uuid
- Parameters
-
[in] uuid the uuid of the node to retrieve [out] id the retrieved id of the node [out] tag the retrieved label of the node [out] description the retrieved description of the node [out] type the retrieved type of the node [out] parentId the retrieved parentId of the node [out] rootId the retrieved rootId of the node [out] created_at timestamp as UNIX epoch when the object was first created [out] modified_at timestamp as UNIX epoch when the object was last modified
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ retrieveObject() [2/2]
|
pure virtual |
retrieves the node based on the id pseudocode for SQL query is SELECT UUID, TAG, DESCRIPTION, TYPE, PARENT, ROOT FROM N WHERE ID = id
- Parameters
-
[in] id the id of the node to retrieve [out] uuid the retrieved uuid of the node [out] tag the retrieved label of the node [out] description the retrieved description of the node [out] type the retrieved type of the node [out] parentId the retrieved parentId of the node [out] rootId the retrieved rootId of the node [out] created_at timestamp as UNIX epoch when the object was first created [out] modified_at timestamp as UNIX epoch when the object was last modified
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ transaction_depth()
int Persistency::transaction_depth | ( | void | ) |
- Returns
- current level of transaction depth; 0 means no transaction is ongoing
◆ try_lock()
|
pure virtual |
try to lock item id, all its descendant objects and all related Q/S/ITBL
- Returns
- true if lock successful, false if not
- Parameters
-
id id in N of item to lock user name of the locking user (input / output: on input, the user attempting the lock, on output unchanged if lock successful, or the user currently holding the lock if unsuccessful) duration time duration in seconds for the lock, after that the lock expires
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ unlock()
|
protected |
◆ update() [1/8]
|
pure virtual |
updates the UPDATED_AT field
- Parameters
-
id the database id of the current Object
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ update() [2/8]
|
pure virtual |
bulk update for Integers; only updates the value; uses an efficient mechanism similar to ODBC's SQLPrepare/ SQLBindParameter / SQLExecute;
- Exceptions
-
Error If one of the items present as second element of the map is not in the I table
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ update() [3/8]
|
pure virtual |
bulk update for IntegerVector; only updates the vector size and the values; uses an efficient mechanism similar to ODBC's SQLPrepare/ SQLBindParameter / SQLExecute;
- Parameters
-
id the database id of the current Object elements collection of IntegerVector variables
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ update() [4/8]
|
pure virtual |
bulk update for Quantities; only updates the value; uses an efficient mechanism similar to ODBC's SQLPrepare/ SQLBindParameter / SQLExecute;
- Exceptions
-
Error If one of the items present as second element of the map is not in the Q table
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ update() [5/8]
|
pure virtual |
bulk update for QuantityMatrix; only updates the matrix size and the values; uses an efficient mechanism similar to ODBC's SQLPrepare/ SQLBindParameter / SQLExecute;
- Parameters
-
id the database id of the current Object elements collection of QuantityMatrix variables
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ update() [6/8]
|
pure virtual |
bulk update for QuantityVectors; only updates the vector size and the values; uses an efficient mechanism similar to ODBC's SQLPrepare/ SQLBindParameter / SQLExecute;
- Parameters
-
id the database id of the current Object elements collection of QuantityVector variables
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ update() [7/8]
|
pure virtual |
bulk update for Strings; only updates the value; uses an efficient mechanism similar to ODBC's SQLPrepare/ SQLBindParameter / SQLExecute;
- Parameters
-
id the database id of the current Object elements collection of Strings variables
- Exceptions
-
Error If one of the items present as second element of the map is not in the Q table
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
◆ update() [8/8]
|
pure virtual |
bulk update for StringVectors; only updates the vector size and the values; uses an efficient mechanism similar to ODBC's SQLPrepare/ SQLBindParameter / SQLExecute;
- Parameters
-
id the database id of the current Object elements collection of StringVectors variables
Implemented in PersistencyOdbc, PersistencyPostgres, and PersistencySqlite.
Member Data Documentation
◆ transaction_depth_
|
protected |
The documentation for this class was generated from the following file: