Manage the Percistency storage inside Postgres data source. More...
#include <PersistencyPostgres.h>
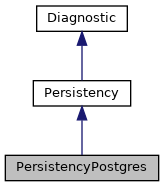
Public Member Functions | |
PersistencyPostgres (void) | |
PersistencyPostgres (const char *conninfo) | |
virtual | ~PersistencyPostgres (void) |
void | purge (const std::string &tablename, int i=-1) override |
void | purgeAll (void) |
override Persistency::purgeAll with more efficient, Postgresql-specific version More... | |
int | countRows (const std::string &tablename, int i=-1) override |
int | getId (void) override |
void | remove (int i, const std::string &tablename) override |
int | countCID (int i, const std::string &tablename) override |
void | push_transaction (void) override |
increment transaction depth More... | |
void | pop_transaction (void) override |
decrement transaction depth; if depth reaches zero, commit More... | |
int | insertObject (const Persistent &object, int offset) override |
void | retrieveObject (int id, std::string &uuid, std::string &tag, std::string &description, std::string &type, int &parentId, int &rootId, double &created_at, double &modified_at) override |
retrieves the node based on the id pseudocode for SQL query is SELECT UUID, TAG, DESCRIPTION, TYPE, PARENT, ROOT FROM N WHERE ID = id More... | |
void | retrieveObject (const std::string &uuid, int &id, std::string &tag, std::string &description, std::string &type, int &parentId, int &rootId, double &created_at, double &modified_at) override |
retrieves the node based on the uuid pseudocode for SQL query is SELECT ID, TAG, DESCRIPTION, TYPE, PARENT, ROOT FROM N WHERE UUID = uuid More... | |
void | retrieveChildren (int id, std::vector< std::pair< int, std::string > > &children) override |
retrieves the node's children based on the id pseudocode for SQL query is SELECT ID, TYPE FROM N WHERE PARENT = id ORDER BY ID More... | |
void | removeObject (int id) override |
remove object from persistency database pseudocode for SQL query is: DELETE FROM {IVV,SVV,QVV,QMV} JOIN {IV,SV,QV,QM} ON IVV.VID = IV.ID WHERE IV.NID IN [id, id+RANGE] DELETE FROM {I,IV,S,SV,Q,QV,QM} WHERE NID IN [id, id+RANGE] DELETE FROM N WHERE ID IN [id, id+RANGE] More... | |
bool | try_lock (int id, std::string &user, int duration) override |
bool | check_lock (int id, std::string &user) override |
bool | release_lock (int id, std::string &user) override |
void | update (int id) override |
void | insert (const std::map< std::string, Integer * > &elements, int offset) override |
void | read (int id, std::map< std::string, Integer * > &elements) override |
void | update (int id, const std::map< std::string, Integer * > &elements) override |
void | insert (const std::map< std::string, Quantity * > &elements, int offset) override |
void | read (int id, std::map< std::string, Quantity * > &elements) override |
void | update (int id, const std::map< std::string, Quantity * > &elements) override |
void | insert (const std::map< std::string, String * > &elements, int offset) override |
void | read (int id, std::map< std::string, String * > &elements) override |
void | update (int id, const std::map< std::string, String * > &elements) override |
void | insert (const std::map< std::string, IntegerVector * > &elements, int offset) override |
void | read (int id, std::map< std::string, IntegerVector * > &elements) override |
void | update (int id, const std::map< std::string, IntegerVector * > &elements) override |
void | insert (const std::map< std::string, QuantityVector * > &elements, int offset) override |
void | read (int id, std::map< std::string, QuantityVector * > &elements) override |
void | update (int id, const std::map< std::string, QuantityVector * > &elements) override |
void | insert (const std::map< std::string, QuantityMatrix * > &elements, int offset) override |
void | read (int id, std::map< std::string, QuantityMatrix * > &elements) override |
void | update (int id, const std::map< std::string, QuantityMatrix * > &elements) override |
void | insert (const std::map< std::string, StringVector * > &elements, int offset) override |
void | read (int id, std::map< std::string, StringVector * > &elements) override |
void | update (int id, const std::map< std::string, StringVector * > &elements) override |
![]() | |
Persistency (void) | |
virtual | ~Persistency (void) |
terminates connection to database More... | |
virtual void | push_transaction (void)=0 |
increment transaction depth More... | |
virtual void | pop_transaction (void)=0 |
decrement transaction depth; if depth reaches zero, commit More... | |
int | transaction_depth (void) |
virtual void | purge (const std::string &tablename, int i=-1)=0 |
void | purgeAll (void) |
purges all tables, N as last More... | |
virtual int | countRows (const std::string &tablename, int i=-1)=0 |
virtual int | getId (void)=0 |
virtual void | remove (int i, const std::string &tablename)=0 |
virtual int | countCID (int i, const std::string &)=0 |
virtual int | insertObject (const Persistent &object, int offset)=0 |
virtual void | retrieveObject (int id, std::string &uuid, std::string &tag, std::string &description, std::string &type, int &parentId, int &rootId, double &created_at, double &modified_at)=0 |
retrieves the node based on the id pseudocode for SQL query is SELECT UUID, TAG, DESCRIPTION, TYPE, PARENT, ROOT FROM N WHERE ID = id More... | |
virtual void | retrieveObject (const std::string &uuid, int &id, std::string &tag, std::string &description, std::string &type, int &parentId, int &rootId, double &created_at, double &modified_at)=0 |
retrieves the node based on the uuid pseudocode for SQL query is SELECT ID, TAG, DESCRIPTION, TYPE, PARENT, ROOT FROM N WHERE UUID = uuid More... | |
virtual void | retrieveChildren (int id, std::vector< std::pair< int, std::string > > &children)=0 |
retrieves the node's children based on the id pseudocode for SQL query is SELECT ID, TYPE FROM N WHERE PARENT = id ORDER BY ID More... | |
virtual void | removeObject (int id)=0 |
remove object from persistency database pseudocode for SQL query is: DELETE FROM {IVV,SVV,QVV,QMV} JOIN {IV,SV,QV,QM} ON IVV.VID = IV.ID WHERE IV.NID IN [id, id+RANGE] DELETE FROM {I,IV,S,SV,Q,QV,QM} WHERE NID IN [id, id+RANGE] DELETE FROM N WHERE ID IN [id, id+RANGE] More... | |
virtual void | update (int id)=0 |
virtual bool | try_lock (int id, std::string &user, int duration)=0 |
virtual bool | check_lock (int id, std::string &user)=0 |
virtual bool | release_lock (int id, std::string &user)=0 |
virtual void | insert (const std::map< std::string, Integer * > &elements, int offset)=0 |
virtual void | read (int id, std::map< std::string, Integer * > &elements)=0 |
virtual void | update (int id, const std::map< std::string, Integer * > &elements)=0 |
int | readInteger (int id, const std::string &tag) |
virtual void | insert (const std::map< std::string, Quantity * > &elements, int offset)=0 |
virtual void | read (int id, std::map< std::string, Quantity * > &elements)=0 |
virtual void | update (int id, const std::map< std::string, Quantity * > &elements)=0 |
virtual void | insert (const std::map< std::string, String * > &elements, int offset)=0 |
virtual void | read (int id, std::map< std::string, String * > &elements)=0 |
virtual void | update (int id, const std::map< std::string, String * > &elements)=0 |
std::string | readString (int id, const std::string &tag) |
virtual void | insert (const std::map< std::string, IntegerVector * > &elements, int offset)=0 |
virtual void | read (int id, std::map< std::string, IntegerVector * > &elements)=0 |
virtual void | update (int id, const std::map< std::string, IntegerVector * > &elements)=0 |
virtual void | insert (const std::map< std::string, QuantityVector * > &elements, int offset)=0 |
virtual void | read (int id, std::map< std::string, QuantityVector * > &elements)=0 |
virtual void | update (int id, const std::map< std::string, QuantityVector * > &elements)=0 |
virtual void | insert (const std::map< std::string, QuantityMatrix * > &elements, int offset)=0 |
virtual void | read (int id, std::map< std::string, QuantityMatrix * > &elements)=0 |
virtual void | update (int id, const std::map< std::string, QuantityMatrix * > &elements)=0 |
virtual void | insert (const std::map< std::string, StringVector * > &elements, int offset)=0 |
virtual void | read (int id, std::map< std::string, StringVector * > &elements)=0 |
virtual void | update (int id, const std::map< std::string, StringVector * > &elements)=0 |
![]() | |
Diagnostic (void) | |
void | setVerbosity (int verbosity) |
sets the verbosity level for this instance More... | |
int | verbosity (void) const |
Additional Inherited Members | |
![]() | |
void | init (void) |
void | destroy (void) |
void | lock (void) |
void | unlock (void) |
![]() | |
virtual | ~Diagnostic ()=default |
![]() | |
int | transaction_depth_ |
![]() | |
int | verbosityInstance |
Detailed Description
Manage the Percistency storage inside Postgres data source.
#include "PersistencyPostgres.h"
- Remarks
- Warning
- Thread safe:
- Extendable:
- Platform dependencies:
Constructor & Destructor Documentation
◆ PersistencyPostgres() [1/2]
PersistencyPostgres::PersistencyPostgres | ( | void | ) |
◆ PersistencyPostgres() [2/2]
|
explicit |
Costructor
- Parameters
-
conninfo name of the Postgres data source/storage
◆ ~PersistencyPostgres()
|
virtual |
Member Function Documentation
◆ check_lock()
|
overridevirtual |
check lock on item id
- Returns
- true if we still hold the lock, false if there is no lock or if somebody else is holding the lock
- Parameters
-
id id in N of item to lock user name of the locking user (input / output: on input, the user attempting the lock, on output unchanged if lock successful, or the user currently holding the lock if unsuccessful)
Implements Persistency.
◆ countCID()
|
overridevirtual |
counts the records with NID == i in table tablename the argument i can only be >=-1 if it is == -1 the function returns the number of records in table tablename The SQL query is: "SELECT COUNT(*) FROM " + tablename if it is >= 0 the function returns the number of records in table tablename with NID equal to the value of the i parameter The SQL query is: "SELECT COUNT(*) FROM " + tablename + " WHERE NID = "+ i Throws an exception if table tablename does not exist.
Implements Persistency.
◆ countRows()
|
overridevirtual |
count rows in table tablename; if i is greater or equal to zero, filter for ID == i the argument i can only be >=-1 if it is == -1 the function returns the number of records in table tablename The SQL query is: SELECT COUNT(*) FROM " + tablename if it is >= 0 the function returns the number of records in table tablename with ID equal to the value of the i parameter; since ID is a primary key for all tables, in this case only two return values are possible: 0 or 1 The SQL query is: "SELECT COUNT(*) FROM " + tablename + " WHERE ID = " + i Throws an exception if table tablename does not exist.
Implements Persistency.
◆ getId()
|
overridevirtual |
- Returns
- the next available id, or 0 if no row is yet present in table N The SQL query is: "SELECT MAX(ID+range-1) FROM N"
- Exceptions
-
Error in case of error
Implements Persistency.
◆ insert() [1/7]
|
overridevirtual |
bulk insert for Integers; uses an efficient mechanism similar to ODBC's SQLPrepare / SQLBindParameter / SQLExecute
- Note
- SQL pseudo-code: insert into I(NID, TAG, DESCRIPTION, VALUE) values(?, ?, ?, ?)
- Parameters
-
elements collection of Integer variables offset offset is added to each parent()->id() to get the NID
Implements Persistency.
◆ insert() [2/7]
|
overridevirtual |
bulk insert for IntegerVectors; uses an efficient mechanism similar to ODBC's SQLPrepare / SQLBindParameter / SQLExecute
- Parameters
-
elements collection of IntegerVector variables offset offset is added to each parent()->id() to get the NID
Implements Persistency.
◆ insert() [3/7]
|
overridevirtual |
bulk insert for Quantities; uses an efficient mechanism similar to ODBC's SQLPrepare / SQLBindParameter / SQLExecute
- Note
- SQL pseudo-code: insert into Q(NID, TAG, DESCRIPTION, VALUE) values(?, ?, ?, ?)
- Parameters
-
elements collection of Quantity variables offset offset is added to each parent()->id() to get the NID
Implements Persistency.
◆ insert() [4/7]
|
overridevirtual |
bulk insert for QuantityMatrix; uses an efficient mechanism similar to ODBC's SQLPrepare / SQLBindParameter / SQLExecute
- Parameters
-
elements collection of QuantityMatrix variables offset offset is added to each parent()->id() to get the NID
Implements Persistency.
◆ insert() [5/7]
|
overridevirtual |
bulk insert for QuantityVectors; uses an efficient mechanism similar to ODBC's SQLPrepare / SQLBindParameter / SQLExecute
- Parameters
-
elements collection of QuantityVector variables offset offset is added to each parent()->id() to get the NID
Implements Persistency.
◆ insert() [6/7]
|
overridevirtual |
bulk insert for Strings; uses an efficient mechanism similar to ODBC's SQLPrepare / SQLBindParameter / SQLExecute
- Note
- SQL pseudo-code: insert into S(NID, TAG, DESCRIPTION, VALUE) values(?, ?, ?, ?)
- Parameters
-
elements collection of String variables offset offset is added to each parent()->id() to get the NID
Implements Persistency.
◆ insert() [7/7]
|
overridevirtual |
bulk insert for StringVectors; uses an efficient mechanism similar to ODBC's SQLPrepare / SQLBindParameter / SQLExecute
- Parameters
-
elements collection of StringVector variables offset offset is added to each parent()->id() to get the NID
Implements Persistency.
◆ insertObject()
|
overridevirtual |
insert object into persistency database SQL Query string: INSERT INTO N (ID, TAG, DESCRIPTION, TYPE, GID, COMPLETETAG, PARENT) VALUES (...)
- Returns
- the database ID of the new object
- Parameters
-
object the item to insert offset offset is added to o.id() and o.parentId() when inserting
Implements Persistency.
◆ pop_transaction()
|
overridevirtual |
decrement transaction depth; if depth reaches zero, commit
Implements Persistency.
◆ purge()
|
overridevirtual |
Deletes all records in the table tablename if i=-1, else a filter is applied see below
- Parameters
-
tablename the name of the table i if tablename == N, delete from N where ID = 1; if tablename == TC delete from TC where finish = i; else delete from tablename where nid = 1 Do nothing if table has no record. Throws an exception if table tablename does not exist. Will be called for the [Q,I,S]TBL tables before so that the relations with the N are enforces at all times even during purging.
Implements Persistency.
◆ purgeAll()
void PersistencyPostgres::purgeAll | ( | void | ) |
override Persistency::purgeAll with more efficient, Postgresql-specific version
◆ push_transaction()
|
overridevirtual |
increment transaction depth
Implements Persistency.
◆ read() [1/7]
|
overridevirtual |
bulk read for Integers; only matching Item's values are updated, if no matching is found a warning is issued
- Note
- SQL pseudo-code: SELECT TAG, VALUE FROM I WHERE NID = id
Implements Persistency.
◆ read() [2/7]
|
overridevirtual |
bulk read for Integers; only matching Item's values are updated, if no matching is found a warning is issued
- Parameters
-
id the database id of the current Object elements collection of IntegerVector variables
Implements Persistency.
◆ read() [3/7]
|
overridevirtual |
bulk read for Quantities; only matching Item's values are updated, if no matching is found a warning is issued
- Note
- SQL pseudo-code: SELECT TAG, VALUE FROM Q WHERE NID = id
Implements Persistency.
◆ read() [4/7]
|
overridevirtual |
bulk read for QuantityMatrix; only matching Item's values are updated, if no matching is found a warning is issued
- Parameters
-
id the database id of the current Object elements collection of QuantityMatrix variables
Implements Persistency.
◆ read() [5/7]
|
overridevirtual |
bulk read for QuantityVectors; only matching Item's values are updated, if no matching is found a warning is issued
- Parameters
-
id the database id of the current Object elements collection of QuantityVector variables
Implements Persistency.
◆ read() [6/7]
|
overridevirtual |
bulk read for Strings; only matching Item's values are updated, if no matching is found a warning is issued
- Note
- SQL pseudo-code: SELECT TAG, VALUE FROM S WHERE NID = id
Implements Persistency.
◆ read() [7/7]
|
overridevirtual |
bulk read for StringVectors; only matching Item's values are updated, if no matching is found a warning is issued
- Parameters
-
id the database id of the current Object elements collection of StringVector variables
Implements Persistency.
◆ release_lock()
|
overridevirtual |
release lock on item id
- Returns
- true if we were still holding the lock, false if not
- Exceptions
-
ErrorRunTime if we hold a lock on a parent object or if the lock cannot be released for any reason
- Parameters
-
id id in N of item to lock user name of the locking user (input / output: on input, the user attempting the lock, on output unchanged if lock successful, or the user currently holding the lock if unsuccessful)
Implements Persistency.
◆ remove()
|
overridevirtual |
removes the record with id == i in table tablename The SQL query is: "DELETE FROM " + tablename + " WHERE ID = " + i Throws an exception if table tablename does not exist.
Implements Persistency.
◆ removeObject()
|
overridevirtual |
remove object from persistency database pseudocode for SQL query is: DELETE FROM {IVV,SVV,QVV,QMV} JOIN {IV,SV,QV,QM} ON IVV.VID = IV.ID WHERE IV.NID IN [id, id+RANGE] DELETE FROM {I,IV,S,SV,Q,QV,QM} WHERE NID IN [id, id+RANGE] DELETE FROM N WHERE ID IN [id, id+RANGE]
- Parameters
-
[in] id the id of the node to retrieve
Implements Persistency.
◆ retrieveChildren()
|
overridevirtual |
retrieves the node's children based on the id pseudocode for SQL query is SELECT ID, TYPE FROM N WHERE PARENT = id ORDER BY ID
- Parameters
-
[in] id the id of the node to retrieve [out] children a vector of child ids and types
Implements Persistency.
◆ retrieveObject() [1/2]
|
overridevirtual |
retrieves the node based on the uuid pseudocode for SQL query is SELECT ID, TAG, DESCRIPTION, TYPE, PARENT, ROOT FROM N WHERE UUID = uuid
- Parameters
-
[in] uuid the uuid of the node to retrieve [out] id the retrieved id of the node [out] tag the retrieved label of the node [out] description the retrieved description of the node [out] type the retrieved type of the node [out] parentId the retrieved parentId of the node [out] rootId the retrieved rootId of the node [out] created_at timestamp as UNIX epoch when the object was first created [out] modified_at timestamp as UNIX epoch when the object was last modified
Implements Persistency.
◆ retrieveObject() [2/2]
|
overridevirtual |
retrieves the node based on the id pseudocode for SQL query is SELECT UUID, TAG, DESCRIPTION, TYPE, PARENT, ROOT FROM N WHERE ID = id
- Parameters
-
[in] id the id of the node to retrieve [out] uuid the retrieved uuid of the node [out] tag the retrieved label of the node [out] description the retrieved description of the node [out] type the retrieved type of the node [out] parentId the retrieved parentId of the node [out] rootId the retrieved rootId of the node [out] created_at timestamp as UNIX epoch when the object was first created [out] modified_at timestamp as UNIX epoch when the object was last modified
Implements Persistency.
◆ try_lock()
|
overridevirtual |
try to lock item id, all its descendant objects and all related Q/S/ITBL
- Returns
- true if lock successful, false if not
- Parameters
-
id id in N of item to lock user name of the locking user (input / output: on input, the user attempting the lock, on output unchanged if lock successful, or the user currently holding the lock if unsuccessful) duration time duration in seconds for the lock, after that the lock expires
Implements Persistency.
◆ update() [1/8]
|
overridevirtual |
updates the UPDATED_AT field
- Parameters
-
id the database id of the current Object
Implements Persistency.
◆ update() [2/8]
|
overridevirtual |
bulk update for Integers; only updates the value; uses an efficient mechanism similar to ODBC's SQLPrepare/ SQLBindParameter / SQLExecute;
- Exceptions
-
Error If one of the items present as second element of the map is not in the I table
Implements Persistency.
◆ update() [3/8]
|
overridevirtual |
bulk update for IntegerVector; only updates the vector size and the values; uses an efficient mechanism similar to ODBC's SQLPrepare/ SQLBindParameter / SQLExecute;
- Parameters
-
id the database id of the current Object elements collection of IntegerVector variables
Implements Persistency.
◆ update() [4/8]
|
overridevirtual |
bulk update for Quantities; only updates the value; uses an efficient mechanism similar to ODBC's SQLPrepare/ SQLBindParameter / SQLExecute;
- Exceptions
-
Error If one of the items present as second element of the map is not in the Q table
Implements Persistency.
◆ update() [5/8]
|
overridevirtual |
bulk update for QuantityMatrix; only updates the matrix size and the values; uses an efficient mechanism similar to ODBC's SQLPrepare/ SQLBindParameter / SQLExecute;
- Parameters
-
id the database id of the current Object elements collection of QuantityMatrix variables
Implements Persistency.
◆ update() [6/8]
|
overridevirtual |
bulk update for QuantityVectors; only updates the vector size and the values; uses an efficient mechanism similar to ODBC's SQLPrepare/ SQLBindParameter / SQLExecute;
- Parameters
-
id the database id of the current Object elements collection of QuantityVector variables
Implements Persistency.
◆ update() [7/8]
|
overridevirtual |
bulk update for Strings; only updates the value; uses an efficient mechanism similar to ODBC's SQLPrepare/ SQLBindParameter / SQLExecute;
- Parameters
-
id the database id of the current Object elements collection of Strings variables
- Exceptions
-
Error If one of the items present as second element of the map is not in the Q table
Implements Persistency.
◆ update() [8/8]
|
overridevirtual |
bulk update for StringVectors; only updates the vector size and the values; uses an efficient mechanism similar to ODBC's SQLPrepare/ SQLBindParameter / SQLExecute;
- Parameters
-
id the database id of the current Object elements collection of StringVectors variables
Implements Persistency.
The documentation for this class was generated from the following file: